Delete a Linked List node at a given position
Last Updated :
22 Feb, 2024
Given a singly linked list and a position, delete a linked list node at the given position.
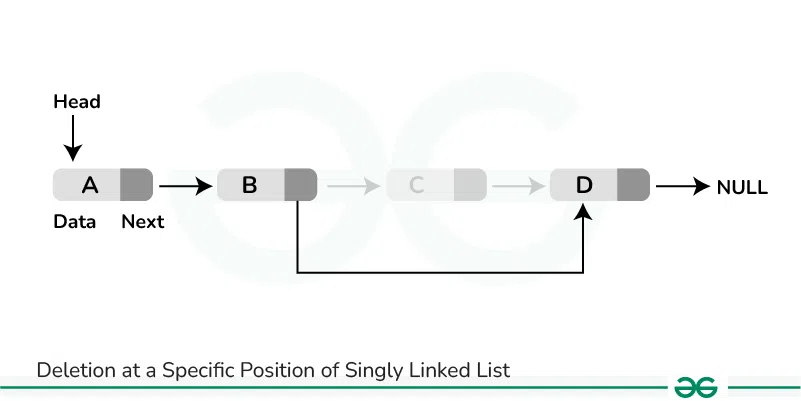
Example:Â Â
Input: position = 1, Linked List = 8->2->3->1->7
Output: Linked List = Â 8->3->1->7
Input: position = 0, Linked List = 8->2->3->1->7
Output: Linked List = 2->3->1->7
Approach:
If the node to be deleted is the root, simply delete it. To delete a middle node, we must have a pointer to the node previous to the node to be deleted.
Here are the algorithmic steps to delete a linked list node at a given position:
- Input: A pointer to the head node of the linked list and the value to be deleted.
- If the linked list is empty, return NULL.
- If the node to be deleted is the head node, set the head node to the next node and delete the original head node.
- Otherwise, traverse the linked list from the head node until the node to be deleted is found.
- If the node to be deleted is not found, return NULL.
- Otherwise, set the previous node’s next pointer to the node after the node to be deleted.
- Delete the node to be deleted.
- Return the head node of the linked list.
Below is the implementation of the above idea.
C++14
#include <iostream>
using namespace std;
class Node {
public :
int data;
Node* next;
};
void push(Node** head_ref, int new_data)
{
Node* new_node = new Node();
new_node->data = new_data;
new_node->next = (*head_ref);
(*head_ref) = new_node;
}
void deleteNode(Node** head_ref, int position)
{
if (*head_ref == NULL)
return ;
Node* temp = *head_ref;
if (position == 0) {
*head_ref = temp->next;
free (temp);
return ;
}
for ( int i = 0; temp != NULL && i < position - 1; i++)
temp = temp->next;
if (temp == NULL || temp->next == NULL)
return ;
Node* next = temp->next->next;
free (temp->next);
temp->next = next;
}
void printList(Node* node)
{
while (node != NULL) {
cout << node->data << " " ;
node = node->next;
}
}
int main()
{
Node* head = NULL;
push(&head, 7);
push(&head, 1);
push(&head, 3);
push(&head, 2);
push(&head, 8);
cout << "Created Linked List: " ;
printList(head);
deleteNode(&head, 4);
cout << "\nLinked List after Deletion at position 4: " ;
printList(head);
return 0;
}
|
C
#include <stdio.h>
#include <stdlib.h>
void insert( int );
void display_List();
void delete ( int );
struct node
{
int data;
struct node* next;
}* head = NULL,
*tail
= NULL;
void delete ( int pos)
{
struct node* temp = head;
int i;
if (pos == 0) {
printf ( "\nElement deleted is : %d\n" , temp->data);
head = head->next;
temp->next = NULL;
free (temp);
}
else {
for (i = 0; i < pos - 1; i++) {
temp = temp->next;
}
struct node* del
= temp->next;
temp->next = temp->next->next;
printf ( "\nElement deleted is : %d\n" , del->data);
del->next = NULL;
free (del);
}
printf ( "\nUpdated Linked List is : \n" );
display_List();
return ;
}
void insert( int value)
{
struct node* newnode;
newnode = ( struct node*) malloc (
sizeof ( struct node));
newnode->data = value;
newnode->next = NULL;
if (head == NULL)
{
head = newnode;
tail = newnode;
}
else
{
tail->next = newnode;
tail = newnode;
}
return ;
}
void display_List()
{
struct node* temp;
temp = head;
while (temp != NULL) {
if (temp->next == NULL) {
printf ( " %d->NULL" , temp->data);
}
else {
printf ( " %d->" , temp->data);
}
temp = temp->next;
}
printf ( "\n" );
return ;
}
int main()
{
insert(10);
insert(20);
insert(30);
insert(40);
insert(50);
insert(60);
printf ( "\n--Created Linked List--\n" );
display_List();
printf ( "\nLinked List after deletion at position 0" );
delete (0);
printf ( "\nLinked List after deletion at position 2" );
delete (2);
return 0;
}
|
Java
import java.io.*;
class LinkedList {
Node head;
class Node {
int data;
Node next;
Node( int d)
{
data = d;
next = null ;
}
}
public void push( int new_data)
{
Node new_node = new Node(new_data);
new_node.next = head;
head = new_node;
}
void deleteNode( int position)
{
if (head == null )
return ;
Node temp = head;
if (position == 0 ) {
head = temp.next;
return ;
}
for ( int i = 0 ; temp != null && i < position - 1 ;
i++)
temp = temp.next;
if (temp == null || temp.next == null )
return ;
Node next = temp.next.next;
temp.next
= next;
}
public void printList()
{
Node tnode = head;
while (tnode != null ) {
System.out.print(tnode.data + " " );
tnode = tnode.next;
}
}
public static void main(String[] args)
{
LinkedList llist = new LinkedList();
llist.push( 7 );
llist.push( 1 );
llist.push( 3 );
llist.push( 2 );
llist.push( 8 );
System.out.println( "\nCreated Linked list is: " );
llist.printList();
llist.deleteNode( 4 );
System.out.println(
"\nLinked List after Deletion at position 4: " );
llist.printList();
}
}
|
Python3
class Node:
def __init__( self , data):
self .data = data
self . next = None
class LinkedList:
def __init__( self ):
self .head = None
def push( self , new_data):
new_node = Node(new_data)
new_node. next = self .head
self .head = new_node
def deleteNodeAtGivenPosition( self , position):
if self .head is None :
return
index = 0
current = self .head
while current. next and index < position:
previous = current
current = current. next
index + = 1
if index < position:
print ( "\nIndex is out of range." )
elif index = = 0 :
self .head = self .head. next
else :
previous. next = current. next
def printList( self ):
temp = self .head
while (temp):
print ( " %d " % (temp.data), end = " " )
temp = temp. next
llist = LinkedList()
llist.push( 7 )
llist.push( 1 )
llist.push( 3 )
llist.push( 2 )
llist.push( 8 )
print ( "Created Linked List: " )
llist.printList()
llist.deleteNodeAtGivenPosition( 4 )
print ( "\nLinked List after Deletion at position 4: " )
llist.printList()
|
C#
using System;
class GFG {
Node head;
public class Node {
public int data;
public Node next;
public Node( int d)
{
data = d;
next = null ;
}
}
public void Push( int new_data)
{
Node new_node = new Node(new_data);
new_node.next = head;
head = new_node;
}
void deleteNode( int position)
{
if (head == null )
return ;
Node temp = head;
if (position == 0) {
head = temp.next;
return ;
}
for ( int i = 0; temp != null && i < position - 1;
i++)
temp = temp.next;
if (temp == null || temp.next == null )
return ;
Node next = temp.next.next;
temp.next = next;
}
public void printList()
{
Node tnode = head;
while (tnode != null ) {
Console.Write(tnode.data + " " );
tnode = tnode.next;
}
}
public static void Main(String[] args)
{
GFG llist = new GFG();
llist.Push(7);
llist.Push(1);
llist.Push(3);
llist.Push(2);
llist.Push(8);
Console.WriteLine( "\nCreated Linked list is: " );
llist.printList();
llist.deleteNode(4);
Console.WriteLine( "\nLinked List after "
+ "Deletion at position 4: " );
llist.printList();
}
}
|
Javascript
<script>
var head;
class Node
{
constructor(val)
{
this .data = val;
this .next = null ;
}
}
function push(new_data)
{
var new_node = new Node(new_data);
new_node.next = head;
head = new_node;
}
function deleteNode(position)
{
if (head == null )
return ;
var temp = head;
if (position == 0)
{
head = temp.next;
return ;
}
for (i = 0; temp != null && i < position - 1; i++)
temp = temp.next;
if (temp == null || temp.next == null )
return ;
var next = temp.next.next;
temp.next = next;
}
function printList()
{
var tnode = head;
while (tnode != null )
{
document.write(tnode.data + " " );
tnode = tnode.next;
}
}
push(7);
push(1);
push(3);
push(2);
push(8);
document.write( "Created Linked list is: <br/>" );
printList();
deleteNode(4);
document.write( "<br/>Linked List after " +
"Deletion at position 4: <br/>" );
printList();
</script>
|
Output
Created Linked List: 8 2 3 1 7
Linked List after Deletion at position 4: 8 2 3 1
Time Complexity:Â
- Best Case: O(1) if given position is 1Â
- Average  & Worst Case: O(N) where N is the length of the linked list. This is because in the worst case, we need to traverse the entire linked list to find the node to be deleted.
Auxiliary Space: O(1) as we are only using a constant amount of extra space for temporary variables and pointers, regardless of the size of the linked list. Specifically, we only need to allocate memory for a few temporary pointers (e.g., current, temp) and the new head pointer if the original head is deleted. The memory for the deleted node(s) is also freed as soon as it is deleted
Like Article
Suggest improvement
Share your thoughts in the comments
Please Login to comment...