Find a triplet such that sum of two equals to third element
Last Updated :
19 Sep, 2023
Given an array of integers, you have to find three numbers such that the sum of two elements equals the third element.
Examples:
Input : {5, 32, 1, 7, 10, 50, 19, 21, 2}
Output : 21, 2, 19
Input : {5, 32, 1, 7, 10, 50, 19, 21, 0}
Output : no such triplet exist
Question source: Arcesium Interview Experience | Set 7 (On campus for Internship)
Simple approach: Run three loops and check if there exists a triplet such that sum of two elements equals the third element.
Code-
C++
#include <bits/stdc++.h>
using namespace std;
void findTriplet( int arr[], int n)
{
for ( int i = 0; i < n; i++) {
for ( int j = i + 1; j < n; j++) {
for ( int k = j + 1; k < n; k++) {
if ((arr[i]+arr[j]==arr[k]) || (arr[i]+arr[k]==arr[j]) || (arr[j]+arr[k]==arr[i])){
cout << "Numbers are: " << arr[i] << " "
<< arr[j] << " " << arr[k];
return ;
}
}
}
}
cout << "No such triplet exists" ;
}
int main()
{
int arr[] = { 5, 32, 1, 7, 10, 50, 19, 21, 2 };
int n = sizeof (arr) / sizeof (arr[0]);
findTriplet(arr, n);
return 0;
}
|
Java
import java.util.*;
public class Main {
public static void findTriplet( int [] arr, int n) {
for ( int i = 0 ; i < n; i++) {
for ( int j = i + 1 ; j < n; j++) {
for ( int k = j + 1 ; k < n; k++) {
if ((arr[i]+arr[j]==arr[k]) || (arr[i]+arr[k]==arr[j]) || (arr[j]+arr[k]==arr[i])) {
System.out.println( "Numbers are: " + arr[i] + " " + arr[j] + " " + arr[k]);
return ;
}
}
}
}
System.out.println( "No such triplet exists" );
}
public static void main(String[] args) {
int [] arr = { 5 , 32 , 1 , 7 , 10 , 50 , 19 , 21 , 2 };
int n = arr.length;
findTriplet(arr, n);
}
}
|
Python3
def findTriplet(arr, n):
for i in range (n):
for j in range (i + 1 , n):
for k in range (j + 1 , n):
if ((arr[i] + arr[j] = = arr[k]) or (arr[i] + arr[k] = = arr[j]) or (arr[j] + arr[k] = = arr[i])):
print ( "Numbers are:" , arr[i], arr[j], arr[k])
return
print ( "No such triplet exists" )
if __name__ = = '__main__' :
arr = [ 5 , 32 , 1 , 7 , 10 , 50 , 19 , 21 , 2 ]
n = len (arr)
findTriplet(arr, n)
|
C#
using System;
public class MainClass {
public static void FindTriplet( int [] arr, int n)
{
for ( int i = 0; i < n; i++) {
for ( int j = i + 1; j < n; j++) {
for ( int k = j + 1; k < n; k++) {
if ((arr[i] + arr[j] == arr[k])
|| (arr[i] + arr[k] == arr[j])
|| (arr[j] + arr[k] == arr[i])) {
Console.WriteLine(
"Numbers are: " + arr[i] + " "
+ arr[j] + " " + arr[k]);
return ;
}
}
}
}
Console.WriteLine( "No such triplet exists" );
}
public static void Main()
{
int [] arr = { 5, 32, 1, 7, 10, 50, 19, 21, 2 };
int n = arr.Length;
FindTriplet(arr, n);
}
}
|
Javascript
function findTriplet(arr) {
const n = arr.length;
for (let i = 0; i < n; i++) {
for (let j = i + 1; j < n; j++) {
for (let k = j + 1; k < n; k++) {
if (
arr[i] + arr[j] === arr[k] ||
arr[i] + arr[k] === arr[j] ||
arr[j] + arr[k] === arr[i]
) {
console.log(`Numbers are: ${arr[i]}, ${arr[j]}, ${arr[k]}`);
return ;
}
}
}
}
console.log( "No such triplet exists" );
}
const arr = [5, 32, 1, 7, 10, 50, 19, 21, 2];
findTriplet(arr);
|
Output
Numbers are: 5 7 2
Time Complexity: O(N^3)
Auxiliary Space: O(1)
Efficient approach: The idea is similar to Find a triplet that sum to a given value.
- Sort the given array first.
- Start fixing the greatest element of three from the back and traverse the array to find the other two numbers which sum up to the third element.
- Take two pointers j(from front) and k(initially i-1) to find the smallest of the two number and from i-1 to find the largest of the two remaining numbers
- If the addition of both the numbers is still less than A[i], then we need to increase the value of the summation of two numbers, thereby increasing the j pointer, so as to increase the value of A[j] + A[k].
- If the addition of both the numbers is more than A[i], then we need to decrease the value of the summation of two numbers, thereby decrease the k pointer so as to decrease the overall value of A[j] + A[k].
Below image is a dry run of the above approach:
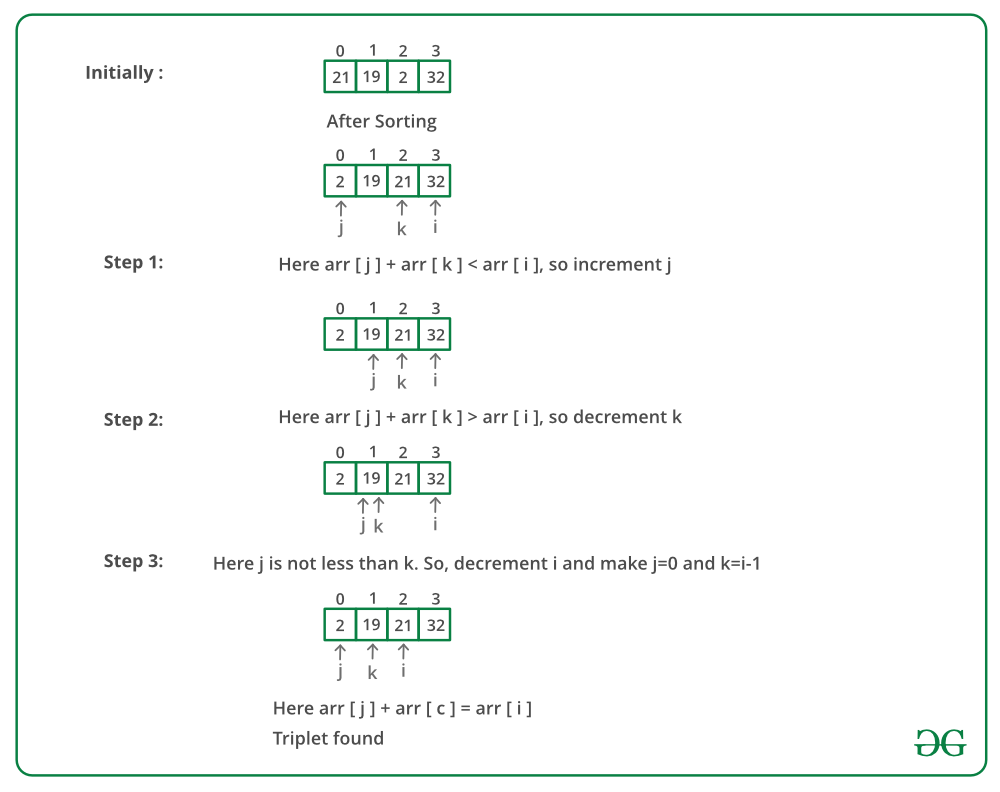
Below is the implementation of the above approach:
C++
#include <bits/stdc++.h>
using namespace std;
void findTriplet( int arr[], int n)
{
sort(arr, arr + n);
for ( int i = n - 1; i >= 0; i--) {
int j = 0;
int k = i - 1;
while (j < k) {
if (arr[i] == arr[j] + arr[k]) {
cout << "numbers are " << arr[i] << " "
<< arr[j] << " " << arr[k] << endl;
return ;
}
else if (arr[i] > arr[j] + arr[k])
j += 1;
else
k -= 1;
}
}
cout << "No such triplet exists" ;
}
int main()
{
int arr[] = { 5, 32, 1, 7, 10, 50, 19, 21, 2 };
int n = sizeof (arr) / sizeof (arr[0]);
findTriplet(arr, n);
return 0;
}
|
Java
import java.util.Arrays;
public class GFG {
static void findTriplet( int arr[], int n)
{
Arrays.sort(arr);
for ( int i = n - 1 ; i >= 0 ; i--) {
int j = 0 ;
int k = i - 1 ;
while (j < k) {
if (arr[i] == arr[j] + arr[k]) {
System.out.println( "numbers are " + arr[i] + " "
+ arr[j] + " " + arr[k]);
return ;
}
else if (arr[i] > arr[j] + arr[k])
j += 1 ;
else
k -= 1 ;
}
}
System.out.println( "No such triplet exists" );
}
public static void main(String args[])
{
int arr[] = { 5 , 32 , 1 , 7 , 10 , 50 , 19 , 21 , 2 };
int n = arr.length;
findTriplet(arr, n);
}
}
|
Python
def findTriplet(arr, n):
arr.sort()
i = n - 1
while (i > = 0 ):
j = 0
k = i - 1
while (j < k):
if (arr[i] = = arr[j] + arr[k]):
print "numbers are " , arr[i], arr[j], arr[k]
return
elif (arr[i] > arr[j] + arr[k]):
j + = 1
else :
k - = 1
i - = 1
print "No such triplet exists"
arr = [ 5 , 32 , 1 , 7 , 10 , 50 , 19 , 21 , 2 ]
n = len (arr)
findTriplet(arr, n)
|
C#
using System;
public class GFG {
static void findTriplet( int [] arr, int n)
{
Array.Sort(arr);
for ( int i = n - 1; i >= 0; i--) {
int j = 0;
int k = i - 1;
while (j < k) {
if (arr[i] == arr[j] + arr[k]) {
Console.WriteLine( "numbers are "
+ arr[i] + " " + arr[j]
+ " " + arr[k]);
return ;
}
else if (arr[i] > arr[j] + arr[k])
j += 1;
else
k -= 1;
}
}
Console.WriteLine( "No such triplet exists" );
}
public static void Main()
{
int [] arr = { 5, 32, 1, 7, 10, 50,
19, 21, 2 };
int n = arr.Length;
findTriplet(arr, n);
}
}
|
PHP
<?php
function findTriplet( $arr , $n )
{
sort( $arr );
for ( $i = $n - 1; $i >= 0; $i --)
{
$j = 0;
$k = $i - 1;
while ( $j < $k )
{
if ( $arr [ $i ] == $arr [ $j ] + $arr [ $k ])
{
echo "numbers are " , $arr [ $i ], " " ,
$arr [ $j ], " " ,
$arr [ $k ];
return ;
}
else if ( $arr [ $i ] > $arr [ $j ] +
$arr [ $k ])
$j += 1;
else
$k -= 1;
}
}
echo "No such triplet exists" ;
}
$arr = array (5, 32, 1, 7, 10,
50, 19, 21, 2 );
$n = count ( $arr );
findTriplet( $arr , $n );
?>
|
Javascript
<script>
function findTriplet(arr, n)
{
arr.sort((a,b) => a-b);
for (let i = n - 1; i >= 0; i--) {
let j = 0;
let k = i - 1;
while (j < k) {
if (arr[i] == arr[j] + arr[k]) {
document.write( "numbers are " + arr[i] +
" " + arr[j] + " " + arr[k] + "<br>" );
return ;
}
else if (arr[i] > arr[j] + arr[k])
j += 1;
else
k -= 1;
}
}
document.write( "No such triplet exists" );
}
let arr = [ 5, 32, 1, 7, 10, 50, 19, 21, 2 ];
let n = arr.length;
findTriplet(arr, n);
</script>
|
Output
numbers are 21 2 19
Time Complexity: O(N^2)
Auxiliary Space: O(1)
Another Approach: The idea is similar to previous approach:
- Sort the given array.
- Start a nested loop, fixing the first element i(from 0 to n-1) and moving the other one j (from i+1 to n-1).
- Take the sum of both the elements and search it in the remaining array using Binary Search.
Implementation:
C++
#include <bits/stdc++.h>
#include <iostream>
using namespace std;
bool search( int sum, int start, int end, int arr[])
{
while (start <= end) {
int mid = (start + end) / 2;
if (arr[mid] == sum) {
return true ;
}
else if (arr[mid] > sum) {
end = mid - 1;
}
else {
start = mid + 1;
}
}
return false ;
}
void findTriplet( int arr[], int n)
{
sort(arr, arr + n);
for ( int i = 0; i < n; i++) {
for ( int j = i + 1; j < n; j++) {
if (search((arr[i] + arr[j]), j, n - 1, arr)) {
cout << "Numbers are: " << arr[i] << " "
<< arr[j] << " " << (arr[i] + arr[j]);
return ;
}
}
}
cout << "No such numbers exist" << endl;
}
int main()
{
int arr[] = { 5, 32, 1, 7, 10, 50, 19, 21, 2 };
int n = sizeof (arr) / sizeof (arr[0]);
findTriplet(arr, n);
return 0;
}
|
Java
import java.util.*;
class GFG{
static boolean search( int sum, int start,
int end, int arr[])
{
while (start <= end)
{
int mid = (start + end) / 2 ;
if (arr[mid] == sum)
{
return true ;
}
else if (arr[mid] > sum)
{
end = mid - 1 ;
}
else
{
start = mid + 1 ;
}
}
return false ;
}
static void findTriplet( int arr[], int n)
{
Arrays.sort(arr);
for ( int i = 0 ; i < n; i++)
{
for ( int j = i + 1 ; j < n; j++)
{
if (search((arr[i] + arr[j]), j, n - 1 , arr))
{
System.out.print( "Numbers are: " + arr[i] + " " +
arr[j] + " " + (arr[i] + arr[j]));
return ;
}
}
}
System.out.print( "No such numbers exist" );
}
public static void main(String args[])
{
int arr[] = { 5 , 32 , 1 , 7 , 10 , 50 , 19 , 21 , 2 };
int n = arr.length;
findTriplet(arr, n);
}
}
|
Python3
from functools import cmp_to_key
def mycmp(a, b):
return a - b
def search( sum , start, end, arr):
while (start < = end):
mid = (start + end) / / 2
if (arr[mid] = = sum ):
return True
elif (arr[mid] > sum ):
end = mid - 1
else :
start = mid + 1
return False
def findTriplet(arr, n):
arr.sort(key = cmp_to_key(mycmp))
for i in range (n):
for j in range (i + 1 ,n):
if (search((arr[i] + arr[j]), j, n - 1 , arr)):
print (f "numbers are {arr[i]} {arr[j]} {( arr[i] + arr[j] )}" )
return
print ( "No such triplet exists" )
arr = [ 5 , 32 , 1 , 7 , 10 , 50 , 19 , 21 , 2 ]
n = len (arr)
findTriplet(arr, n)
|
C#
using System;
public class GFG {
static bool search( int sum, int start, int end, int [] arr)
{
while (start <= end) {
int mid = (start + end) / 2;
if (arr[mid] == sum) {
return true ;
}
else if (arr[mid] > sum) {
end = mid - 1;
}
else {
start = mid + 1;
}
}
return false ;
}
static void findTriplet( int [] arr, int n)
{
Array.Sort(arr);
for ( int i = 0; i < n; i++) {
for ( int j=i+1;j<n;j++)
{
if (search((arr[i] + arr[j]), j, n - 1, arr)) {
Console.WriteLine( "Numbers are "
+ arr[i] + " " + arr[j]
+ " " + (arr[i]+arr[j]));
return ;
}
}
}
Console.WriteLine( "No such triplet exists" );
}
public static void Main()
{
int [] arr = { 5, 32, 1, 7, 10, 50,
19, 21, 2 };
int n = arr.Length;
findTriplet(arr, n);
}
}
|
Javascript
<script>
bool search(sum, start, end, arr)
{
while (start <= end) {
let mid = (start + end) / 2;
if (arr[mid] == sum) {
return true ;
}
else if (arr[mid] > sum) {
end = mid - 1;
}
else {
start = mid + 1;
}
}
return false ;
}
function findTriplet(arr, n)
{
arr.sort((a,b) => a-b);
for (let i = 0; i < n; i++) {
for (let j = i + 1; j < n; j++) {
if (search((arr[i] + arr[j]), j, n - 1, arr)) {
document.write( "numbers are " + arr[i] +
" " + arr[j] + " " + ( arr[i] + arr[j] ) + "<br>" );
}
}
}
document.write( "No such triplet exists" );
}
let arr = [ 5, 32, 1, 7, 10, 50, 19, 21, 2 ];
let n = arr.length;
findTriplet(arr, n);
</script>
|
Output
Numbers are: 2 5 7
Time Complexity: O(N^2*log N)
Auxiliary Space: O(1)
Like Article
Suggest improvement
Share your thoughts in the comments
Please Login to comment...