Sort an array of 0s, 1s and 2s | Dutch National Flag problem
Last Updated :
08 Dec, 2023
Given an array A[] consisting of only 0s, 1s, and 2s. The task is to write a function that sorts the given array. The functions should put all 0s first, then all 1s and all 2s in last.
This problem is also the same as the famous “Dutch National Flag problem”. The problem was proposed by Edsger Dijkstra. The problem is as follows:
Given N balls of colour red, white or blue arranged in a line in random order. You have to arrange all the balls such that the balls with the same colours are adjacent with the order of the balls, with the order of the colours being red, white and blue (i.e., all red coloured balls come first then the white coloured balls and then the blue coloured balls).Â
Examples:
Input: {0, 1, 2, 0, 1, 2}
Output: {0, 0, 1, 1, 2, 2}
Input: {0, 1, 1, 0, 1, 2, 1, 2, 0, 0, 0, 1}
Output: {0, 0, 0, 0, 0, 1, 1, 1, 1, 1, 2, 2}
Sort an array of 0s, 1s, and 2s using the Pointer Approach:Â
This approach is based on the following idea:
- The problem is similar to “Segregate 0s and 1s in an array”.
- The problem was posed with three colors, here `0′, `1′ and `2′. The array is divided into four sections:Â
- arr[1] to arr[low – 1]
- arr[low] to arr[mid – 1]
- arr[mid] to arr[high – 1]
- arr[high] to arr[n]
- If the ith element is 0 then swap the element to the low range.
- Similarly, if the element is 1 then keep it as it is.
- If the element is 2 then swap it with an element in high range.
Illustration:
arr[] = {0, 1, 2, 0, 1, 2}
lo = 0, mid = 0, hi = 5
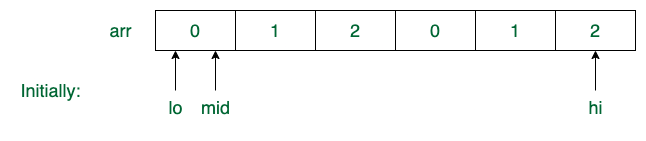
Step – 1: arr[mid] == 0
- swap(arr[lo], arr[mid])
- lo = lo + 1 = 1
- mid = mid + 1 = 1
- arr[] = {0, 1, 2, 0, 1, 2}
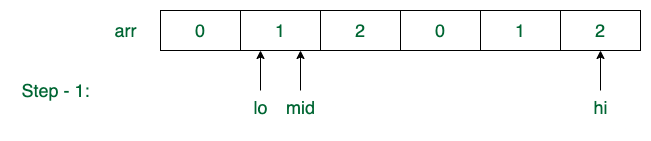
Step – 2: arr[mid] == 1
- mid = mid + 1 = 2
- arr[] = {0, 1, 2, 0, 1, 2}
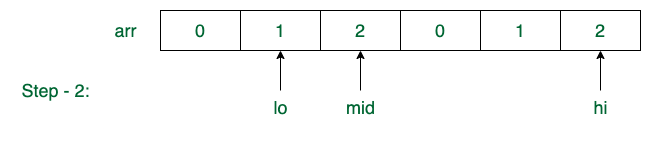
Step – 3: arr[mid] == 2
- swap(arr[mid], arr[hi])
- hi = hi – 1 = 4
- arr[] = {0, 1, 2, 0, 1, 2}
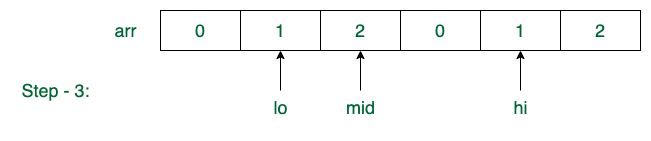
Step – 4: arr[mid] == 2
- swap(arr[mid], arr[hi])
- hi = hi – 1 = 3
- arr[] = {0, 1, 1, 0, 2, 2}
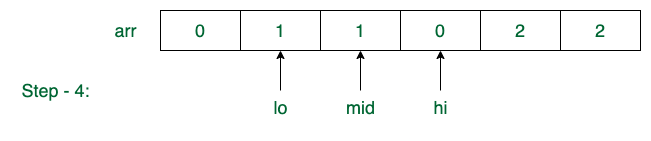
Step – 5: arr[mid] == 1
- mid = mid + 1 = 3
- arr[] = {0, 1, 1, 0, 2, 2}
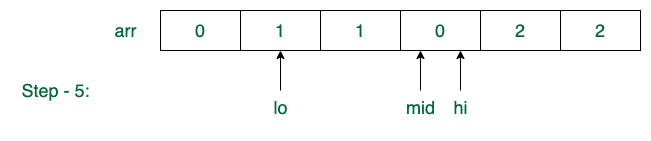
Step – 6: arr[mid] == 0
- swap(arr[lo], arr[mid])
- lo = lo + 1 = 2
- mid = mid + 1 = 4
- arr[] = {0, 0, 1, 1, 2, 2}
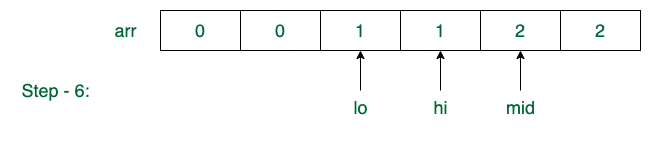
Hence, arr[] = {0, 0, 1, 1, 2, 2}
Follow the steps below to solve the given problem:
- Keep three indices low = 1, mid = 1, and high = N and there are four ranges, 1 to low (the range containing 0), low to mid (the range containing 1), mid to high (the range containing unknown elements) and high to N (the range containing 2).
- Traverse the array from start to end and mid is less than high. (Loop counter is i)
- If the element is 0 then swap the element with the element at index low and update low = low + 1 and mid = mid + 1
- If the element is 1 then update mid = mid + 1
- If the element is 2 then swap the element with the element at index high and update high = high – 1 and update i = i – 1. As the swapped element is not processed
- Print the array.
C++
#include <bits/stdc++.h>
using namespace std;
void sort012( int a[], int arr_size)
{
int lo = 0;
int hi = arr_size - 1;
int mid = 0;
while (mid <= hi) {
switch (a[mid]) {
case 0:
swap(a[lo++], a[mid++]);
break ;
case 1:
mid++;
break ;
case 2:
swap(a[mid], a[hi--]);
break ;
}
}
}
void printArray( int arr[], int arr_size)
{
for ( int i = 0; i < arr_size; i++)
cout << arr[i] << " " ;
}
int main()
{
int arr[] = { 0, 1, 1, 0, 1, 2, 1, 2, 0, 0, 0, 1 };
int n = sizeof (arr) / sizeof (arr[0]);
sort012(arr, n);
printArray(arr, n);
return 0;
}
|
C
#include <stdio.h>
void swap( int * a, int * b);
void sort012( int a[], int arr_size)
{
int lo = 0;
int hi = arr_size - 1;
int mid = 0;
while (mid <= hi) {
switch (a[mid]) {
case 0:
swap(&a[lo++], &a[mid++]);
break ;
case 1:
mid++;
break ;
case 2:
swap(&a[mid], &a[hi--]);
break ;
}
}
}
void swap( int * a, int * b)
{
int temp = *a;
*a = *b;
*b = temp;
}
void printArray( int arr[], int arr_size)
{
int i;
for (i = 0; i < arr_size; i++)
printf ( "%d " , arr[i]);
}
int main()
{
int arr[] = { 0, 1, 1, 0, 1, 2, 1, 2, 0, 0, 0, 1 };
int arr_size = sizeof (arr) / sizeof (arr[0]);
int i;
sort012(arr, arr_size);
printArray(arr, arr_size);
getchar ();
return 0;
}
|
Java
import java.io.*;
class countzot {
static void sort012( int a[], int arr_size)
{
int lo = 0 ;
int hi = arr_size - 1 ;
int mid = 0 , temp = 0 ;
while (mid <= hi) {
switch (a[mid]) {
case 0 : {
temp = a[lo];
a[lo] = a[mid];
a[mid] = temp;
lo++;
mid++;
break ;
}
case 1 :
mid++;
break ;
case 2 : {
temp = a[mid];
a[mid] = a[hi];
a[hi] = temp;
hi--;
break ;
}
}
}
}
static void printArray( int arr[], int arr_size)
{
int i;
for (i = 0 ; i < arr_size; i++)
System.out.print(arr[i] + " " );
System.out.println( "" );
}
public static void main(String[] args)
{
int arr[] = { 0 , 1 , 1 , 0 , 1 , 2 , 1 , 2 , 0 , 0 , 0 , 1 };
int arr_size = arr.length;
sort012(arr, arr_size);
printArray(arr, arr_size);
}
}
|
Python3
def sort012(a, arr_size):
lo = 0
hi = arr_size - 1
mid = 0
while mid < = hi:
if a[mid] = = 0 :
a[lo], a[mid] = a[mid], a[lo]
lo = lo + 1
mid = mid + 1
elif a[mid] = = 1 :
mid = mid + 1
else :
a[mid], a[hi] = a[hi], a[mid]
hi = hi - 1
return a
def printArray(a):
for k in a:
print (k, end = ' ' )
arr = [ 0 , 1 , 1 , 0 , 1 , 2 , 1 , 2 , 0 , 0 , 0 , 1 ]
arr_size = len (arr)
arr = sort012(arr, arr_size)
printArray(arr)
|
C#
using System;
class GFG {
static void sort012( int [] a, int arr_size)
{
int lo = 0;
int hi = arr_size - 1;
int mid = 0, temp = 0;
while (mid <= hi) {
switch (a[mid]) {
case 0: {
temp = a[lo];
a[lo] = a[mid];
a[mid] = temp;
lo++;
mid++;
break ;
}
case 1:
mid++;
break ;
case 2: {
temp = a[mid];
a[mid] = a[hi];
a[hi] = temp;
hi--;
break ;
}
}
}
}
static void printArray( int [] arr, int arr_size)
{
int i;
for (i = 0; i < arr_size; i++)
Console.Write(arr[i] + " " );
Console.WriteLine( "" );
}
public static void Main()
{
int [] arr = { 0, 1, 1, 0, 1, 2, 1, 2, 0, 0, 0, 1 };
int arr_size = arr.Length;
sort012(arr, arr_size);
printArray(arr, arr_size);
}
}
|
Javascript
<script>
function sort012(a,arr_size)
{
let lo = 0;
let hi = arr_size - 1;
let mid = 0;
let temp = 0;
while (mid <= hi)
{
if (a[mid] == 0)
{
temp = a[lo];
a[lo] = a[mid];
a[mid] = temp;
lo++;
mid++;
}
else if (a[mid] == 1)
{
mid++;
}
else
{
temp = a[mid];
a[mid] = a[hi];
a[hi] = temp;
hi--;
}
}
}
function printArray(arr,arr_size)
{
let i;
for (i = 0; i < arr_size; i++)
{
document.write(arr[i] + " " );
}
document.write( "<br>" );
}
let arr= [0, 1, 1, 0, 1, 2, 1, 2, 0, 0, 0, 1 ];
let arr_size = arr.length;
sort012(arr, arr_size);
printArray(arr, arr_size);
</script>
|
PHP
<?php
function sort012(& $a , $arr_size )
{
$lo = 0;
$hi = $arr_size - 1;
$mid = 0;
while ( $mid <= $hi )
{
switch ( $a [ $mid ])
{
case 0:
swap( $a [ $lo ++], $a [ $mid ++]);
break ;
case 1:
$mid ++;
break ;
case 2:
swap( $a [ $mid ], $a [ $hi --]);
break ;
}
}
}
function swap(& $a , & $b )
{
$temp = $a ;
$a = $b ;
$b = $temp ;
}
function printArray(& $arr , $arr_size )
{
for ( $i = 0; $i < $arr_size ; $i ++)
echo $arr [ $i ]. " " ;
echo "\n" ;
}
$arr = array (0, 1, 1, 0, 1, 2,
1, 2, 0, 0, 0, 1);
$arr_size = sizeof( $arr );
sort012( $arr , $arr_size );
printArray( $arr , $arr_size );
?>
|
Output
0 0 0 0 0 1 1 1 1 1 2 2
Time Complexity: O(n), Only one traversal of the array is needed.
Space Complexity: O(1), No extra space is required.
Sort an array of 0s, 1s, and 2s using pointers:
Approach :
- In this approach we will be using two pointers(l and r) along with an iterator(i). The entire array will be divided into three ranges:
- Index 0 to l-1 (range containing 0)
- Index l to r (range containing unknown elements)
- Index r+1 to n (range containing 2)
- Initialise l=0 and r=n-1.
- Inside a for loop, make sure i<=r and do the following steps:
- If the i-th element is 0, swap it with arr[l] and increment l and i.
- If the i-th element is 2, swap it with arr[r] and decrement r (not i). The loop will automatically check for the next updated value of arr[i].
- If the i-th element is arr[i], simply increment i and continue.
Illustration :
arr[] = {0, 1, 2, 0, 1, 2}
Step 1: l=0, r=5, i=0: arr[0]=0 –> Swap arr[0] with arr[0]. l=l+1 and i= i+1.
arr[] = {0, 1, 2, 0, 1, 2 }
Step 2: l=1, r=5, i=1: arr[1]=0 –> i= i+1.
arr[] = {0, 1, 2, 0, 1, 2 }
Step 3: l=1, r=5, i=2: arr[2]=2 –> Swap arr[2] with arr[5]. r=r-1.
arr[] = {0, 1, 2, 0, 1, 2 }
Step 4: l=1, r=4, i=2: arr[2]=2 –> Swap arr[2] with arr[4]. r=r-1.
arr[] = {0, 1, 1, 0, 2, 2 }
Step 5: l=1, r=3, i=2: arr[2]=1 –> i= i+1.
arr[] = {0, 1, 1, 0, 2, 2 }
Step 6: l=1, r=3, i=3: arr[3]=0 –> Swap arr[3] with arr[1]. l=l+1 and i= i+1.
arr[] = {0, 0, 1, 1, 2, 2 }
Follow the given steps to solve the problem :
- Initialise l=0 and r=n-1.
- Inside a for loop, make sure i<=r and do the following steps:
- If the i-th element is 0, swap it with arr[l] and increment l and i.
- If the i-th element is 2, swap it with arr[r] and decrement r (not i). The loop will automatically check for the next updated value of arr[i].
- If the i-th element is arr[i], simply increment i and continue.
C++
#include <iostream>
using namespace std;
void sort012( int arr[], int n)
{
int l=0;
int r=n-1;
for ( int i=0;i<n && i<=r;){
if (arr[i]==0){
swap(arr[l],arr[i]);
l++;
i++;
}
else if (arr[i]==2){
swap(arr[i],arr[r]);
r--;
}
else {
i++;
}
}
}
void printArray( int arr[], int arr_size)
{
for ( int i = 0; i < arr_size; i++)
cout << arr[i] << " " ;
}
int main()
{
int arr[] = { 0, 1, 1, 0, 1, 2, 1, 2, 0, 0, 0, 1 };
int n = sizeof (arr) / sizeof (arr[0]);
sort012(arr, n);
printArray(arr, n);
return 0;
}
|
Java
import java.util.Arrays;
public class Sort012 {
static void sort012( int [] arr, int n) {
int low = 0 ;
int high = n - 1 ;
for ( int i = 0 ; i < n && i <= high;) {
if (arr[i] == 0 ) {
swap(arr, low, i);
low++;
i++;
}
else if (arr[i] == 2 ) {
swap(arr, i, high);
high--;
}
else {
i++;
}
}
}
static void swap( int [] arr, int i, int j) {
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
static void printArray( int arr[], int arr_size) {
for ( int i = 0 ; i < arr_size; i++)
System.out.print(arr[i] + " " );
}
public static void main(String[] args) {
int arr[] = { 0 , 1 , 1 , 0 , 1 , 2 , 1 , 2 , 0 , 0 , 0 , 1 };
int n = arr.length;
sort012(arr, n);
printArray(arr, n);
}
}
|
Python3
def sort012(arr, n):
l = 0
r = n - 1
i = 0
while i < n and i < = r:
if arr[i] = = 0 :
arr[l], arr[i] = arr[i], arr[l]
l + = 1
i + = 1
elif arr[i] = = 2 :
arr[i], arr[r] = arr[r], arr[i]
r - = 1
else :
i + = 1
def printArray(arr, arr_size):
for i in range (arr_size):
print (arr[i], end = " " )
if __name__ = = "__main__" :
arr = [ 0 , 1 , 1 , 0 , 1 , 2 , 1 , 2 , 0 , 0 , 0 , 1 ]
n = len (arr)
sort012(arr, n)
printArray(arr, n)
|
C#
using System;
namespace Sort012
{
class Program
{
static void Sort012( int [] arr, int n)
{
int l = 0;
int r = n - 1;
for ( int i = 0; i < n && i <= r;)
{
if (arr[i] == 0)
{
Swap( ref arr[l], ref arr[i]);
l++;
i++;
}
else if (arr[i] == 2)
{
Swap( ref arr[i], ref arr[r]);
r--;
}
else
{
i++;
}
}
}
static void Swap( ref int a, ref int b)
{
int temp = a;
a = b;
b = temp;
}
static void PrintArray( int [] arr, int arr_size)
{
for ( int i = 0; i < arr_size; i++)
{
Console.Write(arr[i] + " " );
}
}
static void Main( string [] args)
{
int [] arr = { 0, 1, 1, 0, 1, 2, 1, 2, 0, 0, 0, 1 };
int n = arr.Length;
Sort012(arr, n);
PrintArray(arr, n);
}
}
}
|
Javascript
function GFG(arr) {
let l = 0;
let r = arr.length - 1;
for (let i = 0; i < arr.length && i <= r;) {
if (arr[i] === 0) {
[arr[l], arr[i]] = [arr[i], arr[l]];
l++;
i++;
}
else if (arr[i] === 2) {
[arr[i], arr[r]] = [arr[r], arr[i]];
r--;
}
else {
i++;
}
}
}
function printArray(arr) {
for (let i = 0; i < arr.length; i++)
process.stdout.write(arr[i] + " " );
console.log();
}
let arr = [0, 1, 1, 0, 1, 2, 1, 2, 0, 0, 0, 1];
GFG(arr);
printArray(arr);
|
Output
0 0 0 0 0 1 1 1 1 1 2 2
Time Complexity: O(n), we iterate every element only once
Space Complexity: O(1)
Sort an array of 0s, 1s, and 2s using Counting Approach:Â
This approach is based on the following idea:
Count the number of 0s, 1s, and 2s in the given array. Then store all the 0s at the beginning followed by all the 1s and then all the 2s.
Illustration:
arr[] = {0, 1, 2, 0, 1, 2}
cnt0 = 0, cnt1 = 0, cnt2 = 0
At i = 0: arr[0] == 0
At i = 1: arr[1] == 1
At i = 2: arr[2] == 2
At i = 3: arr[3] == 0
At i = 4: arr[4] == 1
At i = 5: arr[5] == 2
Replace cnt0 number of elements with 0 in arr
- arr[] = {0, 0, 2, 0, 1, 2}
Replace cnt1 number of elements with 1 in arr
- arr[] = {0, 0, 1, 1, 1, 2}
Replace cnt2 number of elements with 2 in arr
- arr[] = {0, 0, 1, 1, 2, 2}
Hence, arr[] = {0, 0, 1, 1, 2, 2}
Follow the steps below to solve the given problem: Â
- Keep three counters c0 to count 0s, c1 to count 1s, and c2 to count 2s
- Traverse through the array and increase the count of c0 if the element is 0, increase the count of c1 if the element is 1 and increase the count of c2 if the element is 2
- Now again traverse the array and replace the first c0 elements with 0, the next c1 elements with 1, and the next c2 elements with 2.
Below is the implementation of the above idea :Â
C++
#include <bits/stdc++.h>
using namespace std;
void printArr( int arr[], int n)
{
for ( int i = 0; i < n; i++)
cout << arr[i] << " " ;
}
void sortArr( int arr[], int n)
{
int i, cnt0 = 0, cnt1 = 0, cnt2 = 0;
for (i = 0; i < n; i++) {
switch (arr[i]) {
case 0:
cnt0++;
break ;
case 1:
cnt1++;
break ;
case 2:
cnt2++;
break ;
}
}
i = 0;
while (cnt0 > 0) {
arr[i++] = 0;
cnt0--;
}
while (cnt1 > 0) {
arr[i++] = 1;
cnt1--;
}
while (cnt2 > 0) {
arr[i++] = 2;
cnt2--;
}
printArr(arr, n);
}
int main()
{
int arr[] = { 0, 1, 1, 0, 1, 2, 1, 2, 0, 0, 0, 1 };
int n = sizeof (arr) / sizeof ( int );
sortArr(arr, n);
return 0;
}
|
Java
import java.io.*;
class GFG {
static void printArr( int arr[], int n)
{
for ( int i = 0 ; i < n; i++)
System.out.print(arr[i] + " " );
}
static void sortArr( int arr[], int n)
{
int i, cnt0 = 0 , cnt1 = 0 , cnt2 = 0 ;
for (i = 0 ; i < n; i++) {
switch (arr[i]) {
case 0 :
cnt0++;
break ;
case 1 :
cnt1++;
break ;
case 2 :
cnt2++;
break ;
}
}
i = 0 ;
while (cnt0 > 0 ) {
arr[i++] = 0 ;
cnt0--;
}
while (cnt1 > 0 ) {
arr[i++] = 1 ;
cnt1--;
}
while (cnt2 > 0 ) {
arr[i++] = 2 ;
cnt2--;
}
printArr(arr, n);
}
public static void main(String[] args)
{
int arr[] = { 0 , 1 , 1 , 0 , 1 , 2 , 1 , 2 , 0 , 0 , 0 , 1 };
int n = arr.length;
sortArr(arr, n);
}
}
|
Python3
def printArr(arr, n):
for i in range (n):
print (arr[i], end = " " )
def sortArr(arr, n):
cnt0 = 0
cnt1 = 0
cnt2 = 0
for i in range (n):
if arr[i] = = 0 :
cnt0 + = 1
elif arr[i] = = 1 :
cnt1 + = 1
elif arr[i] = = 2 :
cnt2 + = 1
i = 0
while (cnt0 > 0 ):
arr[i] = 0
i + = 1
cnt0 - = 1
while (cnt1 > 0 ):
arr[i] = 1
i + = 1
cnt1 - = 1
while (cnt2 > 0 ):
arr[i] = 2
i + = 1
cnt2 - = 1
printArr(arr, n)
arr = [ 0 , 1 , 1 , 0 , 1 , 2 , 1 , 2 , 0 , 0 , 0 , 1 ]
n = len (arr)
sortArr(arr, n)
|
C#
using System;
class GFG {
static void printArr( int [] arr, int n)
{
for ( int i = 0; i < n; i++)
Console.Write(arr[i] + " " );
}
static void sortArr( int [] arr, int n)
{
int i, cnt0 = 0, cnt1 = 0, cnt2 = 0;
for (i = 0; i < n; i++) {
switch (arr[i]) {
case 0:
cnt0++;
break ;
case 1:
cnt1++;
break ;
case 2:
cnt2++;
break ;
}
}
i = 0;
while (cnt0 > 0) {
arr[i++] = 0;
cnt0--;
}
while (cnt1 > 0) {
arr[i++] = 1;
cnt1--;
}
while (cnt2 > 0) {
arr[i++] = 2;
cnt2--;
}
printArr(arr, n);
}
public static void Main()
{
int [] arr = { 0, 1, 1, 0, 1, 2, 1, 2, 0, 0, 0, 1 };
int n = arr.Length;
sortArr(arr, n);
}
}
|
Javascript
<script>
function printArr( arr, n)
{
for (let i = 0; i < n; i++)
document.write(arr[i] + " " );
}
function sortArr( arr, n)
{
let i, cnt0 = 0, cnt1 = 0, cnt2 = 0;
for (i = 0; i < n; i++) {
switch (arr[i]) {
case 0:
cnt0++;
break ;
case 1:
cnt1++;
break ;
case 2:
cnt2++;
break ;
}
}
i = 0;
while (cnt0 > 0) {
arr[i++] = 0;
cnt0--;
}
while (cnt1 > 0) {
arr[i++] = 1;
cnt1--;
}
while (cnt2 > 0) {
arr[i++] = 2;
cnt2--;
}
printArr(arr, n);
}
let arr = [0, 1, 1, 0, 1, 2, 1,
2, 0, 0, 0, 1];
let n = arr.length;
sortArr(arr, n);
</script>
|
Output
0 0 0 0 0 1 1 1 1 1 2 2
Time Complexity: O(n), Only nonnested traversals of the array are needed.
Space Complexity: O(1)
Like Article
Suggest improvement
Share your thoughts in the comments
Please Login to comment...