Print all subsequences of a string
Last Updated :
31 Jul, 2023
Given a string, we have to find out all its subsequences of it. A String is a subsequence of a given String, that is generated by deleting some character of a given string without changing its order.
Examples:
Input : abc
Output : a, b, c, ab, bc, ac, abc
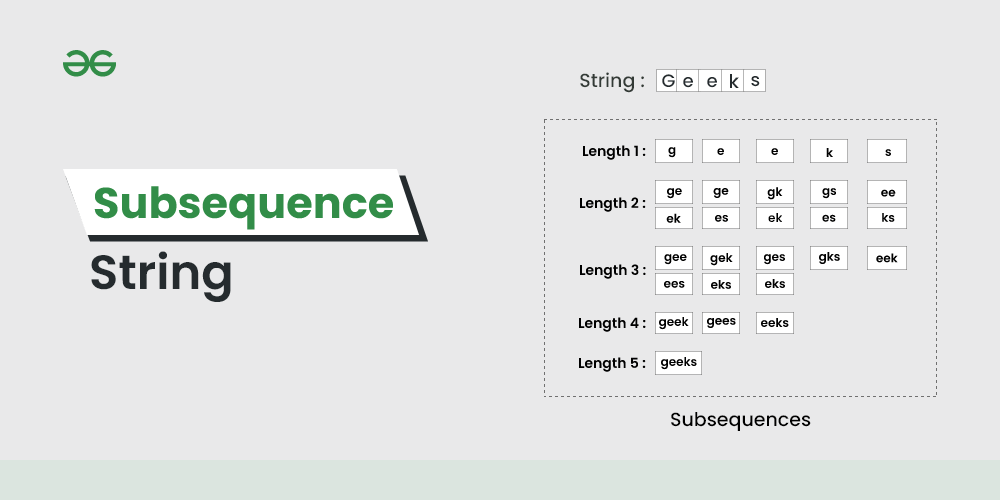
Input : aaa
Output : a, a, a, aa, aa, aa, aaa
Method 1 (Pick and Don’t Pick Concept) :
Implementation:
C++
#include <bits/stdc++.h>
using namespace std;
void printSubsequence(string input, string output)
{
if (input.empty()) {
cout << output << endl;
return ;
}
printSubsequence(input.substr(1), output + input[0]);
printSubsequence(input.substr(1), output);
}
int main()
{
string output = "" ;
string input = "abcd" ;
printSubsequence(input, output);
return 0;
}
|
Java
import java.util.*;
class GFG {
static List<String> al = new ArrayList<>();
public static void main(String[] args)
{
String s = "abcd" ;
findsubsequences(s, "" );
System.out.println(al);
}
private static void findsubsequences(String s,
String ans)
{
if (s.length() == 0 ) {
al.add(ans);
return ;
}
findsubsequences(s.substring( 1 ), ans + s.charAt( 0 ));
findsubsequences(s.substring( 1 ), ans);
}
}
|
Python3
def printSubsequence( input , output):
if len ( input ) = = 0 :
print (output, end = ' ' )
return
printSubsequence( input [ 1 :], output + input [ 0 ])
printSubsequence( input [ 1 :], output)
output = ""
input = "abcd"
printSubsequence( input , output)
|
C#
using System;
using System.Collections.Generic;
class GFG{
static void printSubsequence( string input,
string output)
{
if (input.Length == 0)
{
Console.WriteLine(output);
return ;
}
printSubsequence(input.Substring(1),
output + input[0]);
printSubsequence(input.Substring(1),
output);
}
static void Main()
{
string output = "" ;
string input = "abcd" ;
printSubsequence(input, output);
}
}
|
Javascript
<script>
function printSubsequence(input, output)
{
if (input.length==0) {
document.write( output + "<br>" );
return ;
}
printSubsequence(input.substring(1), output + input[0]);
printSubsequence(input.substring(1), output);
}
var output = "" ;
var input = "abcd" ;
printSubsequence(input, output);
</script>
|
Output
abcd
abc
abd
ab
acd
ac
ad
a
bcd
bc
bd
b
cd
c
d
Time Complexity: O(2^n)
The time complexity of this approach is O(2^n), where n is the length of the given string. This is because, for a string of length n, we are generating a total of 2^n subsequences.
Space Complexity: O(n)
The recursive function call stack requires O(n) space for the worst case, where n is the length of the given string.
Method 2:
Note: This method does not handle duplicate characters.
Explanation :
Step 1: Iterate over the entire String
Step 2: Iterate from the end of string
in order to generate different substring
add the substring to the list
Step 3: Drop kth character from the substring obtained
from above to generate different subsequence.
Step 4: if the subsequence is not in the list then recur.
Below is the implementation of the approach.
C++
#include <bits/stdc++.h>
using namespace std;
unordered_set<string> st;
void subsequence(string str)
{
for ( int i = 0; i < str.length(); i++) {
for ( int j = str.length(); j > i; j--) {
string sub_str = str.substr(i, j);
st.insert(sub_str);
for ( int k = 1; k < sub_str.length(); k++) {
string sb = sub_str;
sb.erase(sb.begin() + k);
subsequence(sb);
}
}
}
}
int main()
{
string s = "aabc" ;
subsequence(s);
for ( auto i : st)
cout << i << " " ;
cout << endl;
return 0;
}
|
Java
import java.util.HashSet;
public class Subsequence {
static HashSet<String> st = new HashSet<>();
static void subsequence(String str)
{
for ( int i = 0 ; i < str.length(); i++) {
for ( int j = str.length(); j > i; j--) {
String sub_str = str.substring(i, j);
if (!st.contains(sub_str))
st.add(sub_str);
for ( int k = 1 ; k < sub_str.length() - 1 ;
k++) {
StringBuffer sb
= new StringBuffer(sub_str);
sb.deleteCharAt(k);
if (!st.contains(sb))
;
subsequence(sb.toString());
}
}
}
}
public static void main(String[] args)
{
String s = "aabc" ;
subsequence(s);
System.out.println(st);
}
}
|
Python3
st = set ()
def subsequence( str ):
for i in range ( len ( str )):
for j in range ( len ( str ),i, - 1 ):
sub_str = str [i: i + j]
st.add(sub_str)
for k in range ( 1 , len (sub_str)):
sb = sub_str
sb = sb.replace(sb[k],"")
subsequence(sb)
s = "aabc"
subsequence(s)
for i in st:
print (i,end = " " )
print ()
|
C#
using System;
using System.Collections.Generic;
using System.Text;
public class GFG {
public static HashSet< string > st
= new HashSet< string >();
public static void subsequence( string str)
{
for ( int i = 0; i < str.Length; i++) {
for ( int j = str.Length; j > i; j--) {
string sub_str = str.Substring(i, j - i);
st.Add(sub_str);
for ( int k = 1; k < sub_str.Length; k++) {
string sb = sub_str;
StringBuilder strBuilder
= new StringBuilder(sb);
strBuilder.Remove(k, 1);
sb = strBuilder.ToString();
subsequence(sb);
}
}
}
}
static public void Main()
{
string s = "aabc" ;
subsequence(s);
foreach ( var value in st)
{
Console.Write(value);
Console.Write( " " );
}
}
}
|
Javascript
<script>
let st = new Set();
function subsequence(str)
{
for (let i = 0; i < str.length; i++) {
for (let j = str.length; j > i; j--) {
let sub_str = str.substr(i, i+j);
st.add(sub_str);
for (let k = 1; k < sub_str.length; k++) {
let sb = sub_str;
sb =sb.replace(sb[k], "" );
subsequence(sb);
}
}
}
}
let s = "aabc" ;
subsequence(s);
for (let i of st)
document.write(i, " " );
document.write( "</br>" );
</script>
|
Output
aab aa aac bc b abc aabc ab ac a c
Time Complexity: O(N^3)
Auxiliary Space: O(N)
Method 3: One by one fix characters and recursively generate all subsets starting from them. After every recursive call, we remove the last character so that the next permutation can be generated.
Implementation:
C++
#include <bits/stdc++.h>
using namespace std;
void printSubSeqRec(string str, int n, int index = -1,
string curr = "" )
{
if (index == n)
return ;
if (!curr.empty()) {
cout << curr << "\n" ;
}
for ( int i = index + 1; i < n; i++) {
curr += str[i];
printSubSeqRec(str, n, i, curr);
curr = curr.erase(curr.size() - 1);
}
return ;
}
void printSubSeq(string str)
{
printSubSeqRec(str, str.size());
}
int main()
{
string str = "cab" ;
printSubSeq(str);
return 0;
}
|
Java
class GFG {
static void printSubSeqRec(String str, int n, int index,
String curr)
{
if (index == n) {
return ;
}
if (curr != null && !curr.trim().isEmpty()) {
System.out.println(curr);
}
for ( int i = index + 1 ; i < n; i++) {
curr += str.charAt(i);
printSubSeqRec(str, n, i, curr);
curr = curr.substring( 0 , curr.length() - 1 );
}
}
static void printSubSeq(String str)
{
int index = - 1 ;
String curr = "" ;
printSubSeqRec(str, str.length(), index, curr);
}
public static void main(String[] args)
{
String str = "cab" ;
printSubSeq(str);
}
}
|
Python3
def printSubSeqRec( str , n, index = - 1 , curr = ""):
if (index = = n):
return
if ( len (curr) > 0 ):
print (curr)
i = index + 1
while (i < n):
curr = curr + str [i]
printSubSeqRec( str , n, i, curr)
curr = curr[ 0 : - 1 ]
i = i + 1
def printSubSeq( str ):
printSubSeqRec( str , len ( str ))
str = "cab"
printSubSeq( str )
|
C#
using System;
public class GFG
{
public static void printSubSeqRec(String str, int n, int index, String curr)
{
if (index == n)
{
return ;
}
if (curr != null && !(curr.Trim().Length == 0))
{
Console.WriteLine(curr);
}
for ( int i = index + 1; i < n; i++)
{
curr += str[i];
GFG.printSubSeqRec(str, n, i, curr);
curr = curr.Substring(0,curr.Length - 1-0);
}
}
public static void printSubSeq(String str)
{
var index = -1;
var curr = "" ;
GFG.printSubSeqRec(str, str.Length, index, curr);
}
public static void Main(String[] args)
{
var str = "cab" ;
GFG.printSubSeq(str);
}
}
|
Javascript
<script>
function printSubSeqRec(str,n,index = -1,curr = "" )
{
if (index == n)
return ;
if (curr.length>0) {
document.write(curr)
}
for (let i = index + 1; i < n; i++) {
curr += str[i];
printSubSeqRec(str, n, i, curr);
curr = curr.slice(0, - 1);
}
return ;
}
function printSubSeq(str)
{
printSubSeqRec(str, str.length);
}
let str = "cab" ;
printSubSeq(str);
</script>
|
Output
c
ca
cab
cb
a
ab
b
Time Complexity: O(n * 2n), where n is the size of the given string
Auxiliary Space: O(n), due to recursive call stack
Method 4: Using Binary representation of numbers to create Subsequences
String = “abc”
All combinations of abc can be represented by all binary representation from 0 to (2^n – 1) where n is the size of the string . The following representation clears things up.
Note : We can also take zero into consideration which will eventually give us an empty set “” , the only change in code will be starting loop from zero.
001 -> “c”
010 -> “b”
011 -> “bc
100 -> “a”
101 -> “ac”
110 -> “ab”
111 -> “abc”
As you can observe we get unique sub-sequences for every set-bit and thus no 2 combinations can be same as 2 numbers cannot have same binary representation.
Input : “abc”
Output :
a
b
a b
c
a c
b c
a b c
Implementation :
- We take the string as input.
- We declare a vector of strings to store each sub-sequence as a string.
- Each time call the function with 1,2,3 and so on and we only push those indexes in our string whose bit is set and we keep incrementing our index pointer.
- Once we have generated the corresponding sub-sequence for a binary representation we can push this string into our vector of strings.
- Finally, we can print our vector of strings and get the desired output.
Below is the implementation of the above approach:
C++
#include<bits/stdc++.h>
using namespace std;
string print(string s , int i){
int j = 0;
string sub;
while (i>0){
if (i & 1){
sub.push_back(s[j]);
}
j++;
i = i >> 1;
}
return sub;
}
vector<string> createsubsets(string& s){
vector <string> res;
for ( int i = 1 ; i <= ((1 << s.size()) - 1) ; i++){
res.push_back(print(s,i));
}
return res;
}
int main(){
string s = "abc" ;
vector <string> print = createsubsets(s);
for ( int i = 0 ; i < print.size() ; i++){
for ( int j = 0; j < print[i].size(); j++){
cout << print[i][j]<< " " ;
}
cout << endl;
}
return 0;
}
|
Java
import java.util.*;
public class GFG {
public static String print(String s , int i){
int j = 0 ;
String sub = "" ;
while (i> 0 ){
if ((i & 1 ) == 1 ){
sub += s.charAt(j);
}
j++;
i = i>> 1 ;
}
return sub;
}
public static List<String> createsubsets(String s){
List<String> res = new ArrayList<>();
for ( int i = 0 ; i < ( 1 <<s.length()) ; i++){
res.add(print(s,i));
}
return res;
}
public static void main(String args[])
{
String s = "abc" ;
List<String> print = createsubsets(s);
for ( int i = 0 ; i < print.size(); i++) {
for ( int j = 0 ; j < print.get(i).length(); j++) {
System.out.print(print.get(i).charAt(j) + " " );
}
System.out.println();
}
}
}
|
Python3
def print_subset(s, i):
j = 0
sub = ""
while i > 0 :
if i & 1 :
sub + = s[j]
j + = 1
i = i >> 1
return sub
def createsubsets(s):
res = []
for i in range ( 1 , ( 1 << len (s))):
res.append(print_subset(s, i))
return res
if __name__ = = "__main__" :
s = "abc"
subsets = createsubsets(s)
for subset in subsets:
for c in subset:
print (c, end = " " )
print ()
|
C#
using System;
using System.Collections.Generic;
namespace GFG
{
class Program
{
public static string Print( string s, int i)
{
int j = 0;
string sub = "" ;
while (i > 0)
{
if ((i & 1) == 1)
{
sub += s[j];
}
j++;
i = i >> 1;
}
return sub;
}
public static List< string > CreateSubsets( string s)
{
List< string > res = new List< string >();
for ( int i = 0; i < (1 << s.Length); i++)
{
res.Add(Print(s, i));
}
return res;
}
static void Main( string [] args)
{
string s = "abc" ;
List< string > print = CreateSubsets(s);
for ( int i = 0; i < print.Count; i++)
{
for ( int j = 0; j < print[i].Length; j++)
{
Console.Write(print[i][j] + " " );
}
Console.WriteLine();
}
}
}
}
|
Javascript
function printSubset(s, i) {
let j = 0;
let sub = "" ;
while (i > 0) {
if (i & 1) {
sub += s[j];
}
j += 1;
i = i >> 1;
}
return sub;
}
function createSubsets(s) {
let res = [];
for (let i = 1; i < (1 << s.length); i++) {
res.push(printSubset(s, i));
}
return res;
}
const s = "abc" ;
const subsets = createSubsets(s);
for (let subset of subsets) {
for (let c of subset) {
process.stdout.write(c + " " );
}
console.log();
}
|
Output
a
b
a b
c
a c
b c
a b c
Time Complexity: O(n* 2^n)
Auxiliary Space: O(n)
Like Article
Suggest improvement
Share your thoughts in the comments
Please Login to comment...