Minimum number of swaps required to sort an array
Last Updated :
18 Sep, 2023
Given an array of N distinct elements, find the minimum number of swaps required to sort the array.
Examples:
Input: {4, 3, 2, 1}
Output: 2
Explanation: Swap index 0 with 3 and 1 with 2 to form the sorted array {1, 2, 3, 4}
Input: {1, 5, 4, 3, 2}
Output: 2
Approach: To solve the problem follow the below idea:
This can be easily done by visualizing the problem as a graph. We will have N nodes and an edge directed from node i to node j if the element at the i’th index must be present at the j’th index in the sorted array.
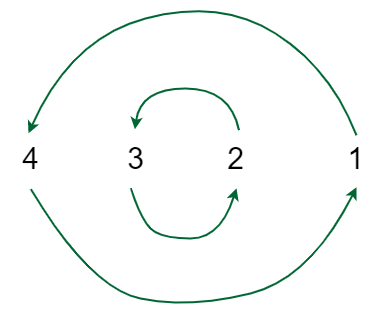
Graph for {4, 3, 2, 1}
The graph will now contain many non-intersecting cycles. Now a cycle with 2 nodes will only require 1 swap to reach the correct ordering, similarly, a cycle with 3 nodes will only require 2 swaps to do so.
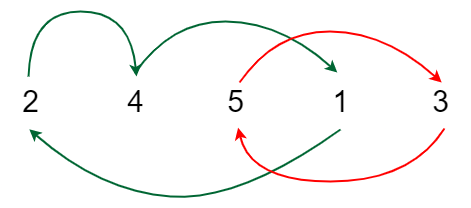
Graph for {2, 4, 5, 1, 3}
Hence, ans = ?i = 1k(cycle_size – 1), where k is the number of cycles
Follow the below steps to solve the problem:
- Create an array of pairs arrPos to store the input array elements along with their index
- Sort arrPos and run a loop for i [0, N]
- If the current element is already visited or it is at its correct position then continue
- Else calculate the cycle size from the current element using a while loop
- Declare an integer j equal to i and in the while loop set j equal to the index of arrPos[j] and increase cycle size while the element at jth position is not visited
- Increase the answer by the size of the current cycle – 1
- Return answer
Below is the implementation of the approach:
C++
#include <bits/stdc++.h>
using namespace std;
int minSwaps( int arr[], int n)
{
pair< int , int > arrPos[n];
for ( int i = 0; i < n; i++) {
arrPos[i].first = arr[i];
arrPos[i].second = i;
}
sort(arrPos, arrPos + n);
vector< bool > vis(n, false );
int ans = 0;
for ( int i = 0; i < n; i++) {
if (vis[i] || arrPos[i].second == i)
continue ;
int cycle_size = 0;
int j = i;
while (!vis[j]) {
vis[j] = 1;
j = arrPos[j].second;
cycle_size++;
}
if (cycle_size > 0) {
ans += (cycle_size - 1);
}
}
return ans;
}
int main()
{
int arr[] = { 1, 5, 4, 3, 2 };
int n = ( sizeof (arr) / sizeof ( int ));
cout << minSwaps(arr, n);
return 0;
}
|
C
#include <stdbool.h>
#include <stdio.h>
#include <stdlib.h>
struct ElementPosition {
int element;
int position;
};
int minSwaps( int arr[], int n)
{
struct ElementPosition arrPos[n];
for ( int i = 0; i < n; i++) {
arrPos[i].element = arr[i];
arrPos[i].position = i;
}
for ( int i = 0; i < n - 1; i++) {
for ( int j = i + 1; j < n; j++) {
if (arrPos[i].element > arrPos[j].element) {
struct ElementPosition temp = arrPos[i];
arrPos[i] = arrPos[j];
arrPos[j] = temp;
}
}
}
bool vis[n];
for ( int i = 0; i < n; i++) {
vis[i] = false ;
}
int ans = 0;
for ( int i = 0; i < n; i++) {
if (vis[i] || arrPos[i].position == i) {
continue ;
}
int cycle_size = 0;
int j = i;
while (!vis[j]) {
vis[j] = true ;
j = arrPos[j].position;
cycle_size++;
}
if (cycle_size > 0) {
ans += (cycle_size - 1);
}
}
return ans;
}
int main()
{
int arr[] = { 1, 5, 4, 3, 2 };
int n = sizeof (arr) / sizeof (arr[0]);
printf ( "%d" , minSwaps(arr, n));
return 0;
}
|
Java
import java.util.*;
import java.util.ArrayList;
import javafx.util.Pair;
class GfG {
public static int minSwaps( int [] arr)
{
int n = arr.length;
ArrayList<Pair<Integer, Integer> > arrpos
= new ArrayList<Pair<Integer, Integer> >();
for ( int i = 0 ; i < n; i++)
arrpos.add(
new Pair<Integer, Integer>(arr[i], i));
arrpos.sort(
new Comparator<Pair<Integer, Integer> >() {
@Override
public int compare(
Pair<Integer, Integer> o1,
Pair<Integer, Integer> o2)
{
if (o1.getKey() > o2.getKey())
return - 1 ;
else if (o1.getKey().equals(
o2.getKey()))
return 0 ;
else
return 1 ;
}
});
Boolean[] vis = new Boolean[n];
Arrays.fill(vis, false );
int ans = 0 ;
for ( int i = 0 ; i < n; i++) {
if (vis[i] || arrpos.get(i).getValue() == i)
continue ;
int cycle_size = 0 ;
int j = i;
while (!vis[j]) {
vis[j] = true ;
j = arrpos.get(j).getValue();
cycle_size++;
}
if (cycle_size > 0 ) {
ans += (cycle_size - 1 );
}
}
return ans;
}
}
class MinSwaps {
public static void main(String[] args)
{
int [] a = { 1 , 5 , 4 , 3 , 2 };
GfG g = new GfG();
System.out.println(g.minSwaps(a));
}
}
|
Python3
def minSwaps(arr):
n = len (arr)
arrpos = [ * enumerate (arr)]
arrpos.sort(key = lambda it: it[ 1 ])
vis = {k: False for k in range (n)}
ans = 0
for i in range (n):
if vis[i] or arrpos[i][ 0 ] = = i:
continue
cycle_size = 0
j = i
while not vis[j]:
vis[j] = True
j = arrpos[j][ 0 ]
cycle_size + = 1
if cycle_size > 0 :
ans + = (cycle_size - 1 )
return ans
arr = [ 1 , 5 , 4 , 3 , 2 ]
print (minSwaps(arr))
|
C#
using System;
using System.Collections.Generic;
using System.Linq;
public class GfG {
public int minSwaps( int [] arr)
{
int n = arr.Length;
List<KeyValuePair< int , int > > arrpos
= new List<KeyValuePair< int , int > >();
for ( int i = 0; i < n; i++)
arrpos.Add(
new KeyValuePair< int , int >(arr[i], i));
arrpos.Sort((a, b) = > a.Key - b.Key);
Boolean[] vis = new Boolean[n];
int ans = 0;
for ( int i = 0; i < n; i++) {
if (vis[i] || arrpos[i].Value == i)
continue ;
int cycle_size = 0;
int j = i;
while (!vis[j]) {
vis[j] = true ;
j = arrpos[j].Value;
cycle_size++;
}
if (cycle_size > 0) {
ans += (cycle_size - 1);
}
}
return ans;
}
}
public class MinSwaps {
public static void Main(String[] args)
{
int [] a = { 1, 5, 4, 3, 2 };
GfG g = new GfG();
Console.WriteLine(g.minSwaps(a));
}
}
|
Javascript
<script>
function minSwaps(arr)
{
let n = arr.length;
let arrpos = [];
for (let i = 0; i < n; i++)
arrpos.push([arr[i], i]);
arrpos.sort( function (a,b){ return a[0]-b[0];});
let vis = new Array(n);
for (let i=0;i<n;i++)
{
vis[i]= false ;
}
let ans = 0;
for (let i = 0; i < n; i++)
{
if (vis[i] || arrpos[i][1] == i)
continue ;
let cycle_size = 0;
let j = i;
while (!vis[j])
{
vis[j] = true ;
j = arrpos[j][1];
cycle_size++;
}
if (cycle_size > 0)
{
ans += (cycle_size - 1);
}
}
return ans;
}
let a=[1, 5, 4, 3, 2];
document.write(minSwaps(a))
</script>
|
Time Complexity: O(N * Log N)
Auxiliary Space: O(N)
Note: As the Pair class is available in java from java 8 so we can use hashmap in the older java version, so instead of using an ArrayList of pairs in java, we can use hashmap, rest of the steps remain the same.
Below is the implementation of the above approach:
C++
#include <bits/stdc++.h>
using namespace std;
int minSwaps( int nums[], int n)
{
int len = n;
map< int , int > map;
for ( int i = 0; i < len; i++)
map[nums[i]] = i;
sort(nums, nums + n);
bool visited[len] = { 0 };
int ans = 0;
for ( int i = 0; i < len; i++) {
if (visited[i] || map[nums[i]] == i)
continue ;
int j = i, cycle_size = 0;
while (!visited[j]) {
visited[j] = true ;
j = map[nums[j]];
cycle_size++;
}
if (cycle_size > 0) {
ans += (cycle_size - 1);
}
}
return ans;
}
int main()
{
int a[] = { 1, 5, 4, 3, 2 };
int n = 5;
cout << minSwaps(a, n);
return 0;
}
|
C
#include <stdio.h>
#include <stdlib.h>
int cmpfunc( const void * a, const void * b)
{
return (*( int *)a - *( int *)b);
}
int minSwaps( int nums[], int n)
{
int len = n;
int map[100001] = { 0 };
for ( int i = 0; i < len; i++)
map[nums[i]] = i;
qsort (nums, n, sizeof ( int ), cmpfunc);
int visited[len];
memset (visited, 0, len * sizeof ( int ));
int ans = 0;
for ( int i = 0; i < len; i++) {
if (visited[i] || map[nums[i]] == i)
continue ;
int j = i, cycle_size = 0;
while (!visited[j]) {
visited[j] = 1;
j = map[nums[j]];
cycle_size++;
}
if (cycle_size > 0) {
ans += (cycle_size - 1);
}
}
return ans;
}
int main()
{
int a[] = { 1, 5, 4, 3, 2 };
int n = 5;
printf ( "%d" , minSwaps(a, n));
return 0;
}
|
Java
import java.io.*;
import java.util.*;
class GfG {
public static int minSwaps( int [] nums)
{
int len = nums.length;
HashMap<Integer, Integer> map = new HashMap<>();
for ( int i = 0 ; i < len; i++)
map.put(nums[i], i);
Arrays.sort(nums);
boolean [] visited = new boolean [len];
Arrays.fill(visited, false );
int ans = 0 ;
for ( int i = 0 ; i < len; i++) {
if (visited[i] || map.get(nums[i]) == i)
continue ;
int j = i, cycle_size = 0 ;
while (!visited[j]) {
visited[j] = true ;
j = map.get(nums[j]);
cycle_size++;
}
if (cycle_size > 0 ) {
ans += (cycle_size - 1 );
}
}
return ans;
}
}
class MinSwaps {
public static void main(String[] args)
{
int [] a = { 1 , 5 , 4 , 3 , 2 };
GfG g = new GfG();
System.out.println(g.minSwaps(a));
}
}
|
Python3
from functools import cmp_to_key
def cmp (a, b):
return a - b
def minSwaps(nums):
Len = len (nums)
map = {}
for i in range ( Len ):
map [nums[i]] = i
nums = sorted (nums, key = cmp_to_key( cmp ))
visited = [ False for col in range ( Len )]
ans = 0
for i in range ( Len ):
if (visited[i] or map [nums[i]] = = i):
continue
j, cycle_size = i, 0
while (visited[j] = = False ):
visited[j] = True
j = map [nums[j]]
cycle_size + = 1
if (cycle_size > 0 ):
ans + = (cycle_size - 1 )
return ans
a = [ 1 , 5 , 4 , 3 , 2 ]
print (minSwaps(a))
|
C#
using System;
using System.Collections.Generic;
class GfG {
public int minSwaps( int [] nums)
{
int len = nums.Length;
Dictionary< int , int > map
= new Dictionary< int , int >();
for ( int i = 0; i < len; i++)
map.Add(nums[i], i);
Array.Sort(nums);
bool [] visited = new bool [len];
int ans = 0;
for ( int i = 0; i < len; i++) {
if (visited[i] || map[nums[i]] == i)
continue ;
int j = i, cycle_size = 0;
while (!visited[j]) {
visited[j] = true ;
j = map[nums[j]];
cycle_size++;
}
if (cycle_size > 0) {
ans += (cycle_size - 1);
}
}
return ans;
}
}
public class MinSwaps {
public static void Main(String[] args)
{
int [] a = { 1, 5, 4, 3, 2 };
GfG g = new GfG();
Console.WriteLine(g.minSwaps(a));
}
}
|
Javascript
<script>
function minSwaps(nums) {
var len = nums.length;
var map = new Map();
for (i = 0; i < len; i++)
map.set(nums[i], i);
nums.sort((a,b)=>a-b);
var visited = Array(len).fill( false );
var ans = 0;
for ( var i = 0; i < len; i++) {
if (visited[i] || map.get(nums[i]) == i)
continue ;
var j = i, cycle_size = 0;
while (!visited[j]) {
visited[j] = true ;
j = map.get(nums[j]);
cycle_size++;
}
if (cycle_size > 0) {
ans += (cycle_size - 1);
}
}
return ans;
}
var a = [ 1, 5, 4, 3, 2 ];
document.write(minSwaps(a));
</script>
|
Time Complexity: O(N Log N)
Auxiliary Space: O(N)
The minimum number of swaps required to sort an array using a greedy algorithm:
To solve the problem follow the below idea:
While iterating over the array, check the current element, and if not in the correct place, replace that element with the index of the element which should have come to this place greedily which will give the optimal answer
Follow the below steps to solve the problem:
- Create a new array and copy the elements of the input array
- Sort the new array and declare a variable ans equal to 0
- Run a for loop to traverse the elements
- If the current element in the sorted array is not equal to the one in the input array then increase the ans by 1
- And swap the current element, with the required element at this index
- Return ans
Below is the implementation of the above approach:
C++
#include <bits/stdc++.h>
using namespace std;
void swap(vector< int >& arr, int i, int j)
{
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
int indexOf(vector< int >& arr, int ele)
{
for ( int i = 0; i < arr.size(); i++) {
if (arr[i] == ele) {
return i;
}
}
return -1;
}
int minSwaps(vector< int > arr, int N)
{
int ans = 0;
vector< int > temp(arr.begin(), arr.end());
sort(temp.begin(), temp.end());
for ( int i = 0; i < N; i++) {
if (arr[i] != temp[i]) {
ans++;
swap(arr, i, indexOf(arr, temp[i]));
}
}
return ans;
}
int main()
{
vector< int > a
= { 101, 758, 315, 730, 472, 619, 460, 479 };
int n = a.size();
cout << minSwaps(a, n);
}
|
C
#include <stdio.h>
#include <stdlib.h>
void swap( int * arr, int i, int j)
{
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
int indexOf( int * arr, int size, int ele)
{
for ( int i = 0; i < size; i++) {
if (arr[i] == ele) {
return i;
}
}
return -1;
}
int cmpfunc( const void * a, const void * b)
{
return (*( int *)a - *( int *)b);
}
int minSwaps( int * arr, int N)
{
int ans = 0;
int * temp = ( int *) malloc ( sizeof ( int ) * N);
for ( int i = 0; i < N; i++) {
temp[i] = arr[i];
}
qsort (temp, N, sizeof ( int ), cmpfunc);
for ( int i = 0; i < N; i++) {
if (arr[i] != temp[i]) {
ans++;
swap(arr, i, indexOf(arr, N, temp[i]));
}
}
return ans;
}
int main()
{
int a[] = { 101, 758, 315, 730, 472, 619, 460, 479 };
int n = sizeof (a) / sizeof (a[0]);
printf ( "%d" , minSwaps(a, n));
return 0;
}
|
Java
import java.io.*;
import java.util.*;
class GfG {
public int minSwaps( int [] arr, int N)
{
int ans = 0 ;
int [] temp = Arrays.copyOfRange(arr, 0 , N);
Arrays.sort(temp);
for ( int i = 0 ; i < N; i++) {
if (arr[i] != temp[i]) {
ans++;
swap(arr, i, indexOf(arr, temp[i]));
}
}
return ans;
}
public void swap( int [] arr, int i, int j)
{
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
public int indexOf( int [] arr, int ele)
{
for ( int i = 0 ; i < arr.length; i++) {
if (arr[i] == ele) {
return i;
}
}
return - 1 ;
}
}
class Main {
public static void main(String[] args) throws Exception
{
int [] a
= { 101 , 758 , 315 , 730 , 472 , 619 , 460 , 479 };
int n = a.length;
System.out.println( new GfG().minSwaps(a, n));
}
}
|
Python3
def minSwaps(arr, N):
ans = 0
temp = arr.copy()
temp.sort()
for i in range (N):
if (arr[i] ! = temp[i]):
ans + = 1
swap(arr, i,
indexOf(arr, temp[i]))
return ans
def swap(arr, i, j):
temp = arr[i]
arr[i] = arr[j]
arr[j] = temp
def indexOf(arr, ele):
for i in range ( len (arr)):
if (arr[i] = = ele):
return i
return - 1
if __name__ = = "__main__" :
a = [ 101 , 758 , 315 , 730 ,
472 , 619 , 460 , 479 ]
n = len (a)
print (minSwaps(a, n))
|
C#
using System;
public class GFG {
static int minSwaps( int [] arr, int N)
{
int ans = 0;
int [] temp = new int [N];
Array.Copy(arr, temp, N);
Array.Sort(temp);
for ( int i = 0; i < N; i++) {
if (arr[i] != temp[i]) {
ans++;
swap(arr, i, indexOf(arr, temp[i]));
}
}
return ans;
}
static void swap( int [] arr, int i, int j)
{
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
static int indexOf( int [] arr, int ele)
{
for ( int i = 0; i < arr.Length; i++) {
if (arr[i] == ele) {
return i;
}
}
return -1;
}
static public void Main()
{
int [] a
= { 101, 758, 315, 730, 472, 619, 460, 479 };
int n = a.Length;
Console.WriteLine(minSwaps(a, n));
}
}
|
Javascript
<script>
function minSwaps(arr,N)
{
let ans = 0;
let temp = [...arr];
temp.sort( function (a,b){ return a-b;});
for (let i = 0; i < N; i++)
{
if (arr[i] != temp[i])
{
ans++;
swap(arr, i, indexOf(arr, temp[i]));
}
}
return ans;
}
function swap(arr,i,j)
{
let temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
function indexOf(arr,ele)
{
for (let i = 0; i < arr.length; i++)
{
if (arr[i] == ele) {
return i;
}
}
return -1;
}
let a=[101, 758, 315, 730, 472,
619, 460, 479 ];
let n = a.length;
document.write(minSwaps(a, n));
</script>
|
Time Complexity: O(N2)
Auxiliary Space: O(N)
Note: We can still improve the complexity by using a hashmap. The main operation here is the indexOf method inside the loop, which costs us n*n. We can improve this section to O(n), by using a hashmap to store the indexes. Still, we use the sort method, so the complexity cannot improve beyond O(n Log n)
The minimum number of swaps required to sort an array using Hash-Map:
Follow the below steps to solve the problem:
- Make a new array (called temp), which is the sorted form of the input array. We know that we need to transform the input array to the new array (temp) in the minimum number of swaps.
- Make a map that stores the elements and their corresponding index, of the input array.
- So at each i starting from 0 to N in the given array, where N is the size of the array:
- If i is not in its correct position according to the sorted array, then
- We will fill this position with the correct element from the hashmap we built earlier. We know the correct element which should come here is temp[i], so we look up the index of this element from the hashmap.
- After swapping the required elements, we update the content of the hashmap accordingly, as temp[i] to the ith position, and arr[i] to where temp[i] was earlier
- Return answer
Below is the implementation of the above approach:
C++
#include <bits/stdc++.h>
using namespace std;
void swap(vector< int >& arr, int i, int j)
{
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
int minSwaps(vector< int > arr, int N)
{
int ans = 0;
vector< int > temp = arr;
map< int , int > h;
sort(temp.begin(), temp.end());
for ( int i = 0; i < N; i++) {
h[arr[i]] = i;
}
for ( int i = 0; i < N; i++) {
if (arr[i] != temp[i]) {
ans++;
int init = arr[i];
swap(arr, i, h[temp[i]]);
h[init] = h[temp[i]];
h[temp[i]] = i;
}
}
return ans;
}
int main()
{
vector< int > a
= { 101, 758, 315, 730, 472, 619, 460, 479 };
int n = a.size();
cout << minSwaps(a, n);
}
|
C
#include <stdio.h>
#include <stdlib.h>
void swap( int * arr, int i, int j)
{
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
int cmpfunc( const void * a, const void * b)
{
return (*( int *)a - *( int *)b);
}
int minSwaps( int arr[], int N)
{
int ans = 0;
int temp[N];
for ( int i = 0; i < N; i++) {
temp[i] = arr[i];
}
int h[N];
for ( int i = 0; i < N; i++) {
h[arr[i]] = i;
}
qsort (temp, N, sizeof ( int ), cmpfunc);
for ( int i = 0; i < N; i++) {
if (arr[i] != temp[i]) {
ans++;
int init = arr[i];
swap(arr, i, h[temp[i]]);
h[init] = h[temp[i]];
h[temp[i]] = i;
}
}
return ans;
}
int main()
{
int a[] = { 101, 758, 315, 730, 472, 619, 460, 479 };
int n = sizeof (a) / sizeof ( int );
printf ( "%d" , minSwaps(a, n));
return 0;
}
|
Java
import java.io.*;
import java.util.*;
class GfG {
public int minSwaps( int [] arr, int N)
{
int ans = 0 ;
int [] temp = Arrays.copyOfRange(arr, 0 , N);
HashMap<Integer, Integer> h
= new HashMap<Integer, Integer>();
Arrays.sort(temp);
for ( int i = 0 ; i < N; i++) {
h.put(arr[i], i);
}
for ( int i = 0 ; i < N; i++) {
if (arr[i] != temp[i]) {
ans++;
int init = arr[i];
swap(arr, i, h.get(temp[i]));
h.put(init, h.get(temp[i]));
h.put(temp[i], i);
}
}
return ans;
}
public void swap( int [] arr, int i, int j)
{
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
class Main {
public static void main(String[] args) throws Exception
{
int [] a
= { 101 , 758 , 315 , 730 , 472 , 619 , 460 , 479 };
int n = a.length;
System.out.println( new GfG().minSwaps(a, n));
}
}
|
Python3
def minSwap(arr, n):
ans = 0
temp = arr.copy()
h = {}
temp.sort()
for i in range (n):
h[arr[i]] = i
init = 0
for i in range (n):
if (arr[i] ! = temp[i]):
ans + = 1
init = arr[i]
arr[i], arr[h[temp[i]]] = arr[h[temp[i]]], arr[i]
h[init] = h[temp[i]]
h[temp[i]] = i
return ans
a = [ 101 , 758 , 315 , 730 ,
472 , 619 , 460 , 479 ]
n = len (a)
print (minSwap(a, n))
|
C#
using System;
using System.Collections.Generic;
using System.Linq;
public class GfG {
public int minSwaps( int [] arr, int N)
{
int ans = 0;
int [] temp = new int [N];
arr.CopyTo(temp, 0);
Dictionary< int , int > h = new Dictionary< int , int >();
Array.Sort(temp);
for ( int i = 0; i < N; i++) {
h.Add(arr[i], i);
}
for ( int i = 0; i < N; i++) {
if (arr[i] != temp[i]) {
ans++;
int init = arr[i];
swap(arr, i, h[temp[i]]);
h[init] = h[temp[i]];
h[temp[i]] = i;
}
}
return ans;
}
public void swap( int [] arr, int i, int j)
{
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
public class GFG {
public static void Main(String[] args)
{
int [] a
= { 101, 758, 315, 730, 472, 619, 460, 479 };
int n = a.Length;
Console.WriteLine( new GfG().minSwaps(a, n));
}
}
|
Javascript
<script>
function swap(arr, i, j)
{
let temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
function minSwaps(arr,N)
{
let ans = 0;
let temp = arr.slice();
let h = new Map();
temp.sort();
for (let i = 0; i < N; i++)
{
h.set(arr[i], i);
}
for (let i = 0; i < N; i++)
{
if (arr[i] != temp[i])
{
ans++;
let init = arr[i];
swap(arr, i, h.get(temp[i]));
h.set(init,h.get(temp[i]));
h.set(temp[i],i);
}
}
return ans;
}
let a = [101, 758, 315, 730, 472, 619, 460, 479];
let n = a.length;
document.write(minSwaps(a, n));
</script>
|
Time Complexity: O(N * Log N)
Auxiliary Space: O(N)
Related Article:
Number of swaps to sort when only adjacent swapping allowed
Like Article
Suggest improvement
Share your thoughts in the comments
Please Login to comment...