How to iterate a Multidimensional Array?
Last Updated :
07 Nov, 2022
Multidimensional arrays are arrays that have more than one dimension. For example, a simple array is a 1-D array, a matrix is a 2-D array, and a cube or cuboid is a 3-D array but how to visualize arrays with more than 3 dimensions, and how to iterate over elements of these arrays? It is simple, just think of any multidimensional array as a collection of arrays of lower dimensions.
n-D array = Collection of (n-1)D arrays
For example, a matrix or 2-D array is a collection of 1-D arrays.
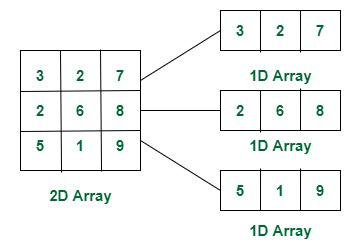
2D Array is a collection of 1D Arrays
Similarly, you can visualize 3-D arrays and other multidimensional arrays.
How to iterate over elements of a Multidimensional array?
It can be observed that only a 1-D array contains elements and a multidimensional array contains smaller dimension arrays.
- Hence first iterate over the smaller dimension array and iterate over the 1-D array inside it.
- Doing this for the whole multidimensional array will iterate over all elements of the multidimensional array.
Example 1: Iterating over a 2-D array
C++
#include <bits/stdc++.h>
using namespace std;
int main()
{
int n = 3;
int m = 3;
int arr[][3]
= { { 3, 2, 7 }, { 2, 6, 8 }, { 5, 1, 9 } };
for ( int i = 0; i < n; i++) {
for ( int j = 0; j < m; j++) {
cout << arr[i][j] << " " ;
}
cout << endl;
}
return 0;
}
|
Java
import java.io.*;
class GFG {
public static void main(String[] args)
{
int n = 3 ;
int m = 3 ;
int [][] arr
= { { 3 , 2 , 7 }, { 2 , 6 , 8 }, { 5 , 1 , 9 } };
for ( int i = 0 ; i < n; i++) {
for ( int j = 0 ; j < m; j++) {
System.out.print(arr[i][j] + " " );
}
System.out.println();
}
}
}
|
Python3
if __name__ = = "__main__" :
n = 3
m = 3
arr = [[ 3 , 2 , 7 ], [ 2 , 6 , 8 ], [ 5 , 1 , 9 ]]
for i in range ( 0 , n):
for j in range ( 0 , m):
print (arr[i][j], end = " " )
print ()
|
C#
using System;
public class GFG{
static public void Main (){
int n = 3;
int m = 3;
int [ , ] arr = { { 3, 2, 7 }, { 2, 6, 8 }, { 5, 1, 9 } };
for ( int i = 0; i < n; i++) {
for ( int j = 0; j < m; j++) {
Console.Write(arr[i,j]);
Console.Write( " " );
}
Console.Write( "\n" );
}
}
}
|
Javascript
<script>
let n = 3;
let m = 3;
let arr
= [[ 3, 2, 7 ], [ 2, 6, 8 ], [ 5, 1, 9 ] ];
for (let i = 0; i < n; i++) {
for (let j = 0; j < m; j++) {
document.write(arr[i][j] + " " );
}
document.write( "<br>" );
}
</script>
|
Time Complexity: O(n*m)
Auxiliary Space: O(1)
Example 2: Iterating over a 3D array
C++
#include <bits/stdc++.h>
using namespace std;
int main()
{
int x = 2, y = 2, z = 2;
int arr[][3][2] = { { { 1, 2 }, { 3, 4 } },
{ { 5, 6 }, { 7, 8 } } };
for ( int i = 0; i < x; i++) {
cout << "Inside " << i + 1
<< " 2D array in 3-D array" << endl;
for ( int j = 0; j < y; j++) {
cout << "Inside " << j + 1
<< " 1D array of the 2-D array" << endl;
for ( int k = 0; k < z; k++) {
cout << arr[i][j][k] << " " ;
}
cout << endl;
}
cout << endl;
}
return 0;
}
|
Java
import java.io.*;
class GFG {
public static void main(String[] args)
{
int x = 2 , y = 2 , z = 2 ;
int [][][] arr = { { { 1 , 2 }, { 3 , 4 } },
{ { 5 , 6 }, { 7 , 8 } } };
for ( int i = 0 ; i < x; i++) {
System.out.println( "Inside " + (i + 1 )
+ " 2D array in 3D array" );
for ( int j = 0 ; j < y; j++) {
System.out.println(
"Inside" + (j + 1 )
+ " 1D array of the 2D array" );
for ( int k = 0 ; k < z; k++) {
System.out.print(arr[i][j][k] + " " );
}
System.out.println();
}
System.out.println();
}
}
}
|
Python3
x = 2
y = 2
z = 2
arr = [ [ [ 1 , 2 ], [ 3 , 4 ] ],[ [ 5 , 6 ], [ 7 , 8 ] ] ]
for i in range ( 0 ,x):
print ( "Inside " + str (i + 1 ) + " 2D array in 3-D array" )
for j in range ( 0 ,x):
print ( "Inside " + str (j + 1 ) + " 1D array of the 2-D array" )
for k in range ( 0 ,x):
print (arr[i][j][k],end = " " )
print ("")
print ("")
|
C#
using System;
public class GFG {
static public void Main()
{
int x = 2, y = 2, z = 2;
int [, , ] arr
= new int [2, 2, 2] { { { 1, 2 }, { 3, 4 } },
{ { 5, 6 }, { 7, 8 } } };
for ( int i = 0; i < x; i++) {
Console.Write( "Inside " + (i + 1)
+ " 2D array in 3D array"
+ "\n" );
for ( int j = 0; j < y; j++) {
Console.Write( "Inside" + (j + 1)
+ " 1D array of the 2D array"
+ "\n" );
for ( int k = 0; k < z; k++) {
Console.Write(arr[i, j, k] + " " );
}
Console.Write( "\n" );
}
Console.Write( "\n" );
}
}
}
|
Javascript
<script>
let x = 2;
let y = 2;
let z = 2;
let arr = [ [ [1, 2 ], [ 3, 4 ] ],
[ [ 5, 6 ], [ 7, 8 ] ] ];
for (let i = 0; i < x; i++) {
document.write( "Inside " + (i + 1)
+ " 2D array in 3-D array" );
document.write( "<br>" );
for (let j = 0; j < y; j++) {
document.write( "Inside " + (j + 1)
+ " 1D array of the 2-D array" );
document.write( "<br>" );
for (let k = 0; k < z; k++) {
document.write( arr[i][j][k] + " " );
}
document.write( "<br>" );
}
document.write( "<br>" );
}
</script>
|
Output
Inside 1 2D array in 3-D array
Inside 1 1D array of the 2-D array
1 2
Inside 2 1D array of the 2-D array
3 4
Inside 2 2D array in 3-D array
Inside 1 1D array of the 2-D array
5 6
Inside 2 1D array of the 2-D array
7 8
Time Complexity: O(x*y*z)
Auxiliary Space: O(1)
Like Article
Suggest improvement
Share your thoughts in the comments
Please Login to comment...