Program to check idempotent matrix
Last Updated :
19 Aug, 2022
Given a N * N matrix and the task is to check matrix is idempotent matrix or not.
Idempotent matrix: A matrix is said to be idempotent matrix if matrix multiplied by itself return the same matrix. The matrix M is said to be idempotent matrix if and only if M * M = M. In idempotent matrix M is a square matrix.
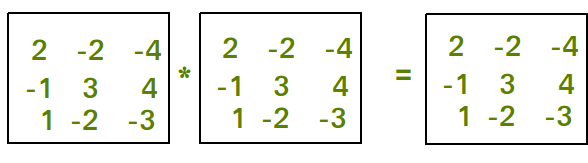
Examples:
Input : mat[][] = {{3, -6},
{1, -2}};
Output : Idempotent Matrix
Input : mat[N][N] = {{2, -2, -4},
{-1, 3, 4},
{1, -2, -3}}
Output : Idempotent Matrix.
Implementation:
C++
#include<bits/stdc++.h>
#define N 3
using namespace std;
void multiply( int mat[][N], int res[][N])
{
for ( int i = 0; i < N; i++)
{
for ( int j = 0; j < N; j++)
{
res[i][j] = 0;
for ( int k = 0; k < N; k++)
res[i][j] += mat[i][k] * mat[k][j];
}
}
}
bool checkIdempotent( int mat[][N])
{
int res[N][N];
multiply(mat, res);
for ( int i = 0; i < N; i++)
for ( int j = 0; j < N; j++)
if (mat[i][j] != res[i][j])
return false ;
return true ;
}
int main()
{
int mat[N][N] = {{2, -2, -4},
{-1, 3, 4},
{1, -2, -3}};
if (checkIdempotent(mat))
cout << "Idempotent Matrix" ;
else
cout << "Not Idempotent Matrix." ;
return 0;
}
|
Java
import java.io.*;
class GFG
{
static int N = 3 ;
static void multiply( int mat[][], int res[][])
{
for ( int i = 0 ; i < N; i++)
{
for ( int j = 0 ; j < N; j++)
{
res[i][j] = 0 ;
for ( int k = 0 ; k < N; k++)
res[i][j] += mat[i][k] * mat[k][j];
}
}
}
static boolean checkIdempotent( int mat[][])
{
int res[][] = new int [N][N];
multiply(mat, res);
for ( int i = 0 ; i < N; i++)
{
for ( int j = 0 ; j < N; j++)
{
if (mat[i][j] != res[i][j])
return false ;
}
}
return true ;
}
public static void main (String[] args)
{
int mat[][] = {{ 2 , - 2 , - 4 },
{- 1 , 3 , 4 },
{ 1 , - 2 , - 3 }};
if (checkIdempotent(mat))
System.out.println( "Idempotent Matrix" );
else
System.out.println( "Not Idempotent Matrix." );
}
}
|
Python 3
import math
def multiply(mat, res):
N = len (mat)
for i in range ( 0 ,N):
for j in range ( 0 ,N):
res[i][j] = 0
for k in range ( 0 ,N):
res[i][j] + = mat[i][k] * mat[k][j]
def checkIdempotent(mat):
N = len (mat)
res = [[ 0 ] * N for i in range ( 0 ,N)]
multiply(mat, res)
for i in range ( 0 ,N):
for j in range ( 0 ,N):
if (mat[i][j] ! = res[i][j]):
return False
return True
mat = [ [ 2 , - 2 , - 4 ],
[ - 1 , 3 , 4 ],
[ 1 , - 2 , - 3 ] ]
if (checkIdempotent(mat)):
print ( "Idempotent Matrix" )
else :
print ( "Not Idempotent Matrix." )
|
C#
using System;
class GFG
{
static int N = 3;
static void multiply( int [,]mat, int [,]res)
{
for ( int i = 0; i < N; i++)
{
for ( int j = 0; j < N; j++)
{
res[i,j] = 0;
for ( int k = 0; k < N; k++)
res[i,j] += mat[i,k] * mat[k,j];
}
}
}
static bool checkIdempotent( int [,]mat)
{
int [,]res = new int [N,N];
multiply(mat, res);
for ( int i = 0; i < N; i++)
{
for ( int j = 0; j < N; j++)
{
if (mat[i,j] != res[i,j])
return false ;
}
}
return true ;
}
public static void Main ()
{
int [,]mat = {{2, -2, 4},
{-1, 3, 4},
{1, -2, -3}};
if (checkIdempotent(mat))
Console.WriteLine( "Idempotent Matrix" );
else
Console.WriteLine( "Not Idempotent Matrix." );
}
}
|
Javascript
<script>
var N = 3;
function multiply(mat, res)
{
for ( var i = 0; i < N; i++)
{
for ( var j = 0; j < N; j++)
{
res[i][j] = 0;
for ( var k = 0; k < N; k++)
res[i][j] += mat[i][k] * mat[k][j];
}
}
return res;
}
function checkIdempotent(mat)
{
var res = Array.from(Array(N), ()=>Array(N).fill(0));
res = multiply(mat, res);
for ( var i = 0; i < N; i++)
{
for ( var j = 0; j < N; j++)
{
if (mat[i][j] != res[i][j])
return false ;
}
}
return true ;
}
var mat = [[2, -2, -4],
[-1, 3, 4],
[1, -2, -3]];
if (checkIdempotent(mat))
document.write( "Idempotent Matrix" );
else
document.write( "Not Idempotent Matrix." );
</script>
|
Time Complexity: O(n3)
Auxiliary Space: O(n2), since n2 extra space has been taken.
Like Article
Suggest improvement
Share your thoughts in the comments
Please Login to comment...