Program for Identity Matrix
Last Updated :
17 Feb, 2023
Introduction to Identity Matrix :
The dictionary definition of an Identity Matrix is a square matrix in which all the elements of the principal or main diagonal are 1’s and all other elements are zeros. In the below image, every matrix is an Identity Matrix.
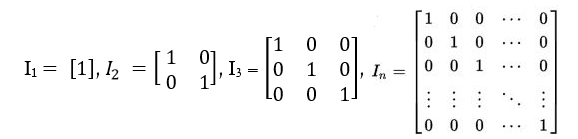
In linear algebra, this is sometimes called as a Unit Matrix, of a square matrix (size = n x n) with ones on the main diagonal and zeros elsewhere. The identity matrix is denoted by “ I “. Sometimes U or E is also used to denote an Identity Matrix.
A property of the identity matrix is that it leaves a matrix unchanged if it is multiplied by an Identity Matrix.
Examples:
Input : 2
Output : 1 0
0 1
Input : 4
Output : 1 0 0 0
0 1 0 0
0 0 1 0
0 0 0 1
The explanation is simple. We need to make all
the elements of principal or main diagonal as
1 and everything else as 0.
Program to print Identity Matrix: The logic is simple. You need to print 1 in those positions where row is equal to the column of a matrix and make all other positions as 0.
Implementation
C++
C
Java
Python3
def Identity(size):
for row in range ( 0 , size):
for col in range ( 0 , size):
if (row = = col):
print ( "1 " , end = " " )
else :
print ( "0 " , end = " " )
print ()
size = 5
Identity(size)
|
C#
PHP
<?php
function Identity( $num )
{
$row ; $col ;
for ( $row = 0; $row < $num ; $row ++)
{
for ( $col = 0; $col < $num ; $col ++)
{
if ( $row == $col )
echo 1, " " ;
else
echo 0, " " ;
}
echo "\n" ;
}
return 0;
}
$size = 5;
identity( $size );
?>
|
Javascript
Output
1 0 0 0 0
0 1 0 0 0
0 0 1 0 0
0 0 0 1 0
0 0 0 0 1
Time Complexity: O(row x col)
Auxiliary Space: O(1), as no extra space is used
Program to check if a given square matrix is Identity Matrix :
C++
#include<iostream>
using namespace std;
const int MAX = 100;
bool isIdentity( int mat[][MAX], int N)
{
for ( int row = 0; row < N; row++)
{
for ( int col = 0; col < N; col++)
{
if (row == col && mat[row][col] != 1)
return false ;
else if (row != col && mat[row][col] != 0)
return false ;
}
}
return true ;
}
int main()
{
int N = 4;
int mat[][MAX] = {{1, 0, 0, 0},
{0, 1, 0, 0},
{0, 0, 1, 0},
{0, 0, 0, 1}};
if (isIdentity(mat, N))
cout << "Yes " ;
else
cout << "No " ;
return 0;
}
|
C
#include <stdio.h>
int isidentity( int a[][100], int N)
{
for ( int i = 0; i < N; i++) {
for ( int j = 0; j < N; j++) {
if (i == j && a[i][j] != 1)
return 0;
else if (i != j && a[i][j] != 0)
return 0;
}
}
return 1;
}
int main()
{
int N = 4;
int a[][100] = { { 1, 0, 0, 0 },
{ 0, 1, 0, 0 },
{ 0, 0, 1, 0 },
{ 0, 0, 0, 1 } };
if (isidentity(a, N))
printf ( "Yes" );
else
printf ( "No" );
return 0;
}
|
Java
import java.io.*;
class GFG {
int MAX = 100 ;
static boolean isIdentity( int mat[][], int N)
{
for ( int row = 0 ; row < N; row++)
{
for ( int col = 0 ; col < N; col++)
{
if (row == col && mat[row][col] != 1 )
return false ;
else if (row != col && mat[row][col] != 0 )
return false ;
}
}
return true ;
}
public static void main(String args[])
{
int N = 4 ;
int mat[][] = {{ 1 , 0 , 0 , 0 },
{ 0 , 1 , 0 , 0 },
{ 0 , 0 , 1 , 0 },
{ 0 , 0 , 0 , 1 }};
if (isIdentity(mat, N))
System.out.println( "Yes " );
else
System.out.println( "No " );
}
}
|
Python3
MAX = 100 ;
def isIdentity(mat, N):
for row in range (N):
for col in range (N):
if (row = = col and
mat[row][col] ! = 1 ):
return False ;
elif (row ! = col and
mat[row][col] ! = 0 ):
return False ;
return True ;
N = 4 ;
mat = [[ 1 , 0 , 0 , 0 ],
[ 0 , 1 , 0 , 0 ],
[ 0 , 0 , 1 , 0 ],
[ 0 , 0 , 0 , 1 ]];
if (isIdentity(mat, N)):
print ( "Yes " );
else :
print ( "No " );
|
C#
using System;
class GFG {
static bool isIdentity( int [,] mat, int N)
{
for ( int row = 0; row < N; row++)
{
for ( int col = 0; col < N; col++)
{
if (row == col && mat[row,col] != 1)
return false ;
else if (row != col && mat[row,col] != 0)
return false ;
}
}
return true ;
}
public static void Main()
{
int N = 4;
int [,]mat = {{1, 0, 0, 0},
{0, 1, 0, 0},
{0, 0, 1, 0},
{0, 0, 0, 1}};
if (isIdentity(mat, N))
Console.WriteLine( "Yes " );
else
Console.WriteLine( "No " );
}
}
|
PHP
<?php
function isIdentity( $mat , $N )
{
for ( $row = 0; $row < $N ; $row ++)
{
for ( $col = 0; $col < $N ; $col ++)
{
if ( $row == $col and
$mat [ $row ][ $col ] != 1)
return false;
else if ( $row != $col &&
$mat [ $row ][ $col ] != 0)
return false;
}
}
return true;
}
$N = 4;
$mat = array ( array (1, 0, 0, 0),
array (0, 1, 0, 0),
array (0, 0, 1, 0),
array (0, 0, 0, 1));
if (isIdentity( $mat , $N ))
echo "Yes " ;
else
echo "No " ;
?>
|
Javascript
<script>
let MAX = 100;
function isIdentity(mat, N)
{
for (let row = 0; row < N; row++)
{
for (let col = 0; col < N; col++)
{
if (row == col && mat[row][col] != 1)
return false ;
else if (row != col && mat[row][col] != 0)
return false ;
}
}
return true ;
}
let N = 4;
let mat = [ [ 1, 0, 0, 0 ],
[ 0, 1, 0, 0 ],
[ 0, 0, 1, 0 ],
[ 0, 0, 0, 1 ] ];
if (isIdentity(mat, N))
document.write( "Yes " );
else
document.write( "No " );
</script>
|
Time Complexity: O(row x col)
Auxiliary Space: O(1), as no extra space is used
Like Article
Suggest improvement
Share your thoughts in the comments
Please Login to comment...