Validity of a given Tic-Tac-Toe board configuration
Last Updated :
11 Aug, 2023
A Tic-Tac-Toe board is given after some moves are played. Find out if the given board is valid, i.e., is it possible to reach this board position after some moves or not.
Note that every arbitrary filled grid of 9 spaces isn’t valid e.g. a grid filled with 3 X and 6 O isn’t valid situation because each player needs to take alternate turns.
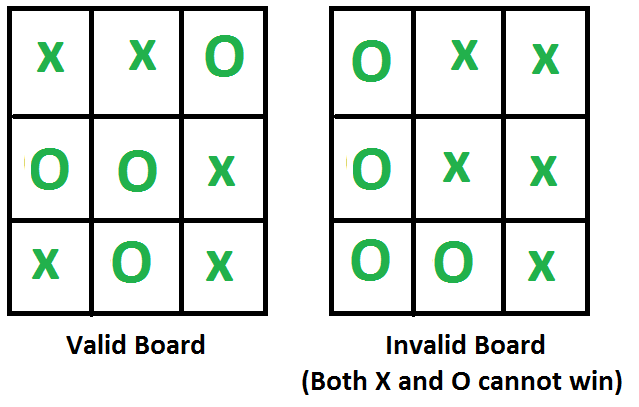
Input is given as a 1D array of size 9.
Examples:
Input: board[] = {'X', 'X', 'O',
'O', 'O', 'X',
'X', 'O', 'X'};
Output: Valid
Input: board[] = {'O', 'X', 'X',
'O', 'X', 'X',
'O', 'O', 'X'};
Output: Invalid
(Both X and O cannot win)
Input: board[] = {'O', 'X', ' ',
' ', ' ', ' ',
' ', ' ', ' '};
Output: Valid
(Valid board with only two moves played)
Basically, to find the validity of an input grid, we can think of the conditions when an input grid is invalid. Let no. of “X”s be countX and no. of “O”s be countO. Since we know that the game starts with X, a given grid of Tic-Tac-Toe game would be definitely invalid if following two conditions meet
- countX != countO AND
- countX != countO + 1
- Since “X” is always the first move, second condition is also required.
- Now does it mean that all the remaining board positions are valid one? The answer is NO. Think of the cases when input grid is such that both X and O are making straight lines. This is also not
- valid position because the game ends when one player wins. So we need to check the following condition as well
- If input grid shows that both the players are in winning situation, it’s an invalid position.
- If input grid shows that the player with O has put a straight-line (i.e. is in win condition) and countX != countO, it’s an invalid position. The reason is that O plays his move only after X plays his
- move. Since X has started the game, O would win when both X and O has played equal no. of moves.
- If input grid shows that X is in winning condition than xCount must be one greater that oCount.
- Armed with above conditions i.e. a), b), c) and d), we can now easily formulate an algorithm/program to check the validity of a given Tic-Tac-Toe board position.
1) countX == countO or countX == countO + 1
2) If O is in win condition then check
a) If X also wins, not valid
b) If xbox != obox , not valid
3) If X is in win condition then check if xCount is
one more than oCount or not
Another way to find the validity of a given board is using ‘inverse method’ i.e. rule out all the possibilities when a given board is invalid.
C++
#include <iostream>
using namespace std;
int win[8][3] = {{0, 1, 2},
{3, 4, 5},
{6, 7, 8},
{0, 3, 6},
{1, 4, 7},
{2, 5, 8},
{0, 4, 8},
{2, 4, 6}};
bool isCWin( char *board, char c)
{
for ( int i=0; i<8; i++)
if (board[win[i][0]] == c &&
board[win[i][1]] == c &&
board[win[i][2]] == c )
return true ;
return false ;
}
bool isValid( char board[9])
{
int xCount=0, oCount=0;
for ( int i=0; i<9; i++)
{
if (board[i]== 'X' ) xCount++;
if (board[i]== 'O' ) oCount++;
}
if (xCount==oCount || xCount==oCount+1)
{
if (isCWin(board, 'O' ))
{
if (isCWin(board, 'X' ))
return false ;
return (xCount == oCount);
}
if (isCWin(board, 'X' ) && xCount != oCount + 1)
return false ;
return true ;
}
return false ;
}
int main()
{
char board[] = { 'X' , 'X' , 'O' ,
'O' , 'O' , 'X' ,
'X' , 'O' , 'X' };
(isValid(board))? cout << "Given board is valid" :
cout << "Given board is not valid" ;
return 0;
}
|
Java
import java.io.*;
class GFG {
static int win[][] = {{ 0 , 1 , 2 },
{ 3 , 4 , 5 },
{ 6 , 7 , 8 },
{ 0 , 3 , 6 },
{ 1 , 4 , 7 },
{ 2 , 5 , 8 },
{ 0 , 4 , 8 },
{ 2 , 4 , 6 }};
static boolean isCWin( char [] board, char c) {
for ( int i = 0 ; i < 8 ; i++) {
if (board[win[i][ 0 ]] == c
&& board[win[i][ 1 ]] == c
&& board[win[i][ 2 ]] == c) {
return true ;
}
}
return false ;
}
static boolean isValid( char board[]) {
int xCount = 0 , oCount = 0 ;
for ( int i = 0 ; i < 9 ; i++) {
if (board[i] == 'X' ) {
xCount++;
}
if (board[i] == 'O' ) {
oCount++;
}
}
if (xCount == oCount || xCount == oCount + 1 ) {
if (isCWin(board, 'O' )) {
if (isCWin(board, 'X' )) {
return false ;
}
return (xCount == oCount);
}
if (isCWin(board, 'X' ) && xCount != oCount + 1 ) {
return false ;
}
return true ;
}
return false ;
}
public static void main(String[] args) {
char board[] = { 'X' , 'X' , 'O' , 'O' , 'O' , 'X' , 'X' , 'O' , 'X' };
if ((isValid(board))) {
System.out.println( "Given board is valid" );
} else {
System.out.println( "Given board is not valid" );
}
}
}
|
Python3
def win_check(arr, char):
matches = [[ 0 , 1 , 2 ], [ 3 , 4 , 5 ],
[ 6 , 7 , 8 ], [ 0 , 3 , 6 ],
[ 1 , 4 , 7 ], [ 2 , 5 , 8 ],
[ 0 , 4 , 8 ], [ 2 , 4 , 6 ]]
for i in range ( 8 ):
if (arr[(matches[i][ 0 ])] = = char and
arr[(matches[i][ 1 ])] = = char and
arr[(matches[i][ 2 ])] = = char):
return True
return False
def is_valid(arr):
xcount = arr.count( 'X' )
ocount = arr.count( 'O' )
if (xcount = = ocount + 1 or xcount = = ocount):
if win_check(arr, 'O' ):
if win_check(arr, 'X' ):
return "Invalid"
if xcount = = ocount:
return "Valid"
if win_check(arr, 'X' ) and xcount ! = ocount + 1 :
return "Invalid"
if not win_check(arr, 'O' ):
return "valid"
return "Invalid"
arr = [ 'X' , 'X' , 'O' ,
'O' , 'O' , 'X' ,
'X' , 'O' , 'X' ]
print ( "Given board is " + is_valid(arr))
|
C#
using System;
class GFG
{
public static int [][] win = new int [][]
{
new int [] {0, 1, 2},
new int [] {3, 4, 5},
new int [] {6, 7, 8},
new int [] {0, 3, 6},
new int [] {1, 4, 7},
new int [] {2, 5, 8},
new int [] {0, 4, 8},
new int [] {2, 4, 6}
};
public static bool isCWin( char [] board,
char c)
{
for ( int i = 0; i < 8; i++)
{
if (board[win[i][0]] == c &&
board[win[i][1]] == c &&
board[win[i][2]] == c)
{
return true ;
}
}
return false ;
}
public static bool isValid( char [] board)
{
int xCount = 0, oCount = 0;
for ( int i = 0; i < 9; i++)
{
if (board[i] == 'X' )
{
xCount++;
}
if (board[i] == 'O' )
{
oCount++;
}
}
if (xCount == oCount ||
xCount == oCount + 1)
{
if (isCWin(board, 'O' ))
{
if (isCWin(board, 'X' ))
{
return false ;
}
return (xCount == oCount);
}
if (isCWin(board, 'X' ) &&
xCount != oCount + 1)
{
return false ;
}
return true ;
}
return false ;
}
public static void Main( string [] args)
{
char [] board = new char [] { 'X' , 'X' , 'O' , 'O' , 'O' ,
'X' , 'X' , 'O' , 'X' };
if ((isValid(board)))
{
Console.WriteLine( "Given board is valid" );
}
else
{
Console.WriteLine( "Given board is not valid" );
}
}
}
|
Javascript
<script>
function isCWin(board, c)
{
let win = new Array( new Array(0, 1, 2),
new Array(3, 4, 5),
new Array(6, 7, 8),
new Array(0, 3, 6),
new Array(1, 4, 7),
new Array(2, 5, 8),
new Array(0, 4, 8),
new Array(2, 4, 6));
for (let i = 0; i < 8; i++)
if (board[win[i][0]] == c &&
board[win[i][1]] == c &&
board[win[i][2]] == c )
return true ;
return false ;
}
function isValid(board)
{
let xCount = 0;
let oCount = 0;
for (let i = 0; i < 9; i++)
{
if (board[i] == 'X' ) xCount++;
if (board[i] == 'O' ) oCount++;
}
if (xCount == oCount || xCount == oCount + 1)
{
if (isCWin(board, 'O' ))
{
if (isCWin(board, 'X' ))
return false ;
return (xCount == oCount);
}
if (isCWin(board, 'X' ) &&
xCount != oCount + 1)
return false ;
return true ;
}
return false ;
}
let board = new Array( 'X' , 'X' , 'O' , 'O' ,
'O' , 'X' , 'X' , 'O' , 'X' );
if (isValid(board))
document.write( "Given board is valid" );
else
document.write( "Given board is not valid" );
</script>
|
PHP
<?php
function isCWin( $board , $c )
{
$win = array ( array (0, 1, 2),
array (3, 4, 5),
array (6, 7, 8),
array (0, 3, 6),
array (1, 4, 7),
array (2, 5, 8),
array (0, 4, 8),
array (2, 4, 6));
for ( $i = 0; $i < 8; $i ++)
if ( $board [ $win [ $i ][0]] == $c &&
$board [ $win [ $i ][1]] == $c &&
$board [ $win [ $i ][2]] == $c )
return true;
return false;
}
function isValid(& $board )
{
$xCount = 0;
$oCount = 0;
for ( $i = 0; $i < 9; $i ++)
{
if ( $board [ $i ] == 'X' ) $xCount ++;
if ( $board [ $i ] == 'O' ) $oCount ++;
}
if ( $xCount == $oCount || $xCount == $oCount + 1)
{
if (isCWin( $board , 'O' ))
{
if (isCWin( $board , 'X' ))
return false;
return ( $xCount == $oCount );
}
if (isCWin( $board , 'X' ) &&
$xCount != $oCount + 1)
return false;
return true;
}
return false;
}
$board = array ( 'X' , 'X' , 'O' , 'O' ,
'O' , 'X' , 'X' , 'O' , 'X' );
if (isValid( $board ))
echo ( "Given board is valid" );
else
echo ( "Given board is not valid" );
?>
|
Output
Given board is valid
Time complexity: O(1)
Auxiliary Space: O(1), since no extra space has been taken.
Approach 2:
The algorithm to check if a Tic-Tac-Toe board is valid or not is as follows:
- Initialize a 2D array win of size 8×3, which contains all possible winning combinations in Tic-Tac-Toe. Each row of the win array represents a winning combination, and each element in a row represents a cell index on the board.
- Define a function isCWin(board, c) which takes a board configuration board and a character c (‘X’ or ‘O’) as inputs, and returns true if character c has won on the board.
- Inside the isCWin function, iterate over each row of the win array. Check if the board has the same character c at all three cell indices of the current row. If yes, return true, as the character c has won.
- Define a function isValid(board) which takes a board configuration board as input, and returns true if the board is valid, else returns false.
- Inside the isValid function, count the number of ‘X’ and ‘O’ characters on the board, and store them in xCount and oCount variables, respectively.
- The board can be valid only if either xCount and oCount are the same, or xCount is one more than oCount.
- If ‘O’ is a winner on the board, check if ‘X’ is also a winner. If yes, return false as both ‘X’ and ‘O’ cannot win at the same time. If not, return true if xCount and oCount are the same, else return false.
- If ‘X’ is a winner on the board, then xCount must be one more than oCount. If not, return false.
- If ‘O’ is not a winner, return true as the board is valid.
Here is the code of the above approach:
C++
#include<bits/stdc++.h>
using namespace std;
bool isWinner( char *board, char c)
{
if ((board[0] == c && board[1] == c && board[2] == c) ||
(board[3] == c && board[4] == c && board[5] == c) ||
(board[6] == c && board[7] == c && board[8] == c) ||
(board[0] == c && board[3] == c && board[6] == c) ||
(board[1] == c && board[4] == c && board[7] == c) ||
(board[2] == c && board[5] == c && board[8] == c) ||
(board[0] == c && board[4] == c && board[8] == c) ||
(board[2] == c && board[4] == c && board[6] == c))
return true ;
return false ;
}
bool isValid( char board[9])
{
int xCount=0, oCount=0;
for ( int i=0; i<9; i++)
{
if (board[i]== 'X' ) xCount++;
if (board[i]== 'O' ) oCount++;
}
if (xCount==oCount || xCount==oCount+1)
{
if (isWinner(board, 'X' ) && isWinner(board, 'O' ))
return false ;
if (isWinner(board, 'X' ) && xCount != oCount + 1)
return false ;
if (isWinner(board, 'O' ) && xCount != oCount)
return false ;
return true ;
}
return false ;
}
int main()
{
char board[] = { 'X' , 'X' , 'O' ,
'O' , 'O' , 'X' ,
'X' , 'O' , 'X' };
(isValid(board))? cout << "Given board is valid" :
cout << "Given board is not valid" ;
return 0;
}
|
Java
import java.util.Arrays;
public class TicTacToe {
public static boolean isWinner( char [] board, char c) {
if ((board[ 0 ] == c && board[ 1 ] == c && board[ 2 ] == c) ||
(board[ 3 ] == c && board[ 4 ] == c && board[ 5 ] == c) ||
(board[ 6 ] == c && board[ 7 ] == c && board[ 8 ] == c) ||
(board[ 0 ] == c && board[ 3 ] == c && board[ 6 ] == c) ||
(board[ 1 ] == c && board[ 4 ] == c && board[ 7 ] == c) ||
(board[ 2 ] == c && board[ 5 ] == c && board[ 8 ] == c) ||
(board[ 0 ] == c && board[ 4 ] == c && board[ 8 ] == c) ||
(board[ 2 ] == c && board[ 4 ] == c && board[ 6 ] == c))
return true ;
return false ;
}
public static boolean isValid( char [] board) {
int xCount = 0 , oCount = 0 ;
for ( int i = 0 ; i < 9 ; i++) {
if (board[i] == 'X' )
xCount++;
if (board[i] == 'O' )
oCount++;
}
if (xCount == oCount || xCount == oCount + 1 ) {
if (isWinner(board, 'X' ) && isWinner(board, 'O' ))
return false ;
if (isWinner(board, 'X' ) && xCount != oCount + 1 )
return false ;
if (isWinner(board, 'O' ) && xCount != oCount)
return false ;
return true ;
}
return false ;
}
public static void main(String[] args) {
char [] board = { 'X' , 'X' , 'O' ,
'O' , 'O' , 'X' ,
'X' , 'O' , 'X' };
if (isValid(board))
System.out.println( "Given board is valid" );
else
System.out.println( "Given board is not valid" );
}
}
|
Python3
def isWinner(board, c):
if (board[ 0 ] = = c and board[ 1 ] = = c and board[ 2 ] = = c) or \
(board[ 3 ] = = c and board[ 4 ] = = c and board[ 5 ] = = c) or \
(board[ 6 ] = = c and board[ 7 ] = = c and board[ 8 ] = = c) or \
(board[ 0 ] = = c and board[ 3 ] = = c and board[ 6 ] = = c) or \
(board[ 1 ] = = c and board[ 4 ] = = c and board[ 7 ] = = c) or \
(board[ 2 ] = = c and board[ 5 ] = = c and board[ 8 ] = = c) or \
(board[ 0 ] = = c and board[ 4 ] = = c and board[ 8 ] = = c) or \
(board[ 2 ] = = c and board[ 4 ] = = c and board[ 6 ] = = c):
return True
return False
def isValid(board):
xCount = 0
oCount = 0
for i in range ( 9 ):
if board[i] = = 'X' :
xCount + = 1
if board[i] = = 'O' :
oCount + = 1
if xCount = = oCount or xCount = = oCount + 1 :
if isWinner(board, 'X' ) and isWinner(board, 'O' ):
return False
if isWinner(board, 'X' ) and xCount ! = oCount + 1 :
return False
if isWinner(board, 'O' ) and xCount ! = oCount:
return False
return True
return False
board = [ 'X' , 'X' , 'O' ,
'O' , 'O' , 'X' ,
'X' , 'O' , 'X' ]
if isValid(board):
print ( "Given board is valid" )
else :
print ( "Given board is not valid" )
|
C#
using System;
public class TicTacToe {
public static bool IsWinner( char [] board, char c) {
if ((board[0] == c && board[1] == c && board[2] == c) ||
(board[3] == c && board[4] == c && board[5] == c) ||
(board[6] == c && board[7] == c && board[8] == c) ||
(board[0] == c && board[3] == c && board[6] == c) ||
(board[1] == c && board[4] == c && board[7] == c) ||
(board[2] == c && board[5] == c && board[8] == c) ||
(board[0] == c && board[4] == c && board[8] == c) ||
(board[2] == c && board[4] == c && board[6] == c))
return true ;
return false ;
}
public static bool IsValid( char [] board) {
int xCount = 0, oCount = 0;
for ( int i = 0; i < 9; i++) {
if (board[i] == 'X' )
xCount++;
if (board[i] == 'O' )
oCount++;
}
if (xCount == oCount || xCount == oCount + 1) {
if (IsWinner(board, 'X' ) && IsWinner(board, 'O' ))
return false ;
if (IsWinner(board, 'X' ) && xCount != oCount + 1)
return false ;
if (IsWinner(board, 'O' ) && xCount != oCount)
return false ;
return true ;
}
return false ;
}
public static void Main( string [] args) {
char [] board = { 'X' , 'X' , 'O' ,
'O' , 'O' , 'X' ,
'X' , 'O' , 'X' };
if (IsValid(board))
Console.WriteLine( "Given board is valid" );
else
Console.WriteLine( "Given board is not valid" );
}
}
|
Javascript
function isWinner(board, c) {
if (
(board[0] === c && board[1] === c && board[2] === c) ||
(board[3] === c && board[4] === c && board[5] === c) ||
(board[6] === c && board[7] === c && board[8] === c) ||
(board[0] === c && board[3] === c && board[6] === c) ||
(board[1] === c && board[4] === c && board[7] === c) ||
(board[2] === c && board[5] === c && board[8] === c) ||
(board[0] === c && board[4] === c && board[8] === c) ||
(board[2] === c && board[4] === c && board[6] === c)
) {
return true ;
}
return false ;
}
function isValid(board) {
let xCount = 0;
let oCount = 0;
for (let i = 0; i < 9; i++) {
if (board[i] === 'X' ) xCount++;
if (board[i] === 'O' ) oCount++;
}
if (xCount == oCount || xCount == oCount + 1) {
if (isWinner(board, 'X' ) && isWinner(board, 'O' )) {
return false ;
}
if (isWinner(board, 'X' ) && xCount !== oCount + 1) {
return false ;
}
if (isWinner(board, 'O' ) && xCount !== oCount) {
return false ;
}
return true ;
}
return false ;
}
const board = [ 'X' , 'X' , 'O' , 'O' , 'O' , 'X' , 'X' , 'O' , 'X' ];
isValid(board) ? console.log( 'Given board is valid' ) : console.log( 'Given board is not valid' );
|
Output
Given board is valid
Time complexity: O(N^2)
Auxiliary Space: O(N)
Thanks to Utkarsh for suggesting this solution. This article is contributed by Aarti_Rathi and Utkarsh.
Like Article
Suggest improvement
Share your thoughts in the comments
Please Login to comment...