Program to find transpose of a matrix
Last Updated :
12 Oct, 2023
Given a matrix of size N X M, find the transpose of the matrix
Transpose of a matrix is obtained by changing rows to columns and columns to rows. In other words, transpose of A[N][M] is obtained by changing A[i][j] to A[j][i].
Example:
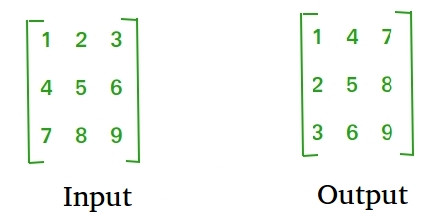
Approach: Follow the given steps to solve the problem:
- Run a nested loop using two integer pointers i and j for 0 <= i < N and 0 <= j < M
- Set B[i][j] equal to A[j][i]
Below is the implementation of the above approach:
C++
#include <bits/stdc++.h>
using namespace std;
#define N 4
void transpose( int A[][N], int B[][N])
{
int i, j;
for (i = 0; i < N; i++)
for (j = 0; j < N; j++)
B[i][j] = A[j][i];
}
int main()
{
int A[N][N] = { { 1, 1, 1, 1 },
{ 2, 2, 2, 2 },
{ 3, 3, 3, 3 },
{ 4, 4, 4, 4 } };
int B[N][N], i, j;
transpose(A, B);
cout << "Result matrix is \n" ;
for (i = 0; i < N; i++) {
for (j = 0; j < N; j++)
cout << " " << B[i][j];
cout << "\n" ;
}
return 0;
}
|
C
#include <stdio.h>
#define N 4
void transpose( int A[][N], int B[][N])
{
int i, j;
for (i = 0; i < N; i++)
for (j = 0; j < N; j++)
B[i][j] = A[j][i];
}
int main()
{
int A[N][N] = { { 1, 1, 1, 1 },
{ 2, 2, 2, 2 },
{ 3, 3, 3, 3 },
{ 4, 4, 4, 4 } };
int B[N][N], i, j;
transpose(A, B);
printf ( "Result matrix is \n" );
for (i = 0; i < N; i++) {
for (j = 0; j < N; j++)
printf ( "%d " , B[i][j]);
printf ( "\n" );
}
return 0;
}
|
Java
class GFG {
static final int N = 4 ;
static void transpose( int A[][], int B[][])
{
int i, j;
for (i = 0 ; i < N; i++)
for (j = 0 ; j < N; j++)
B[i][j] = A[j][i];
}
public static void main(String[] args)
{
int A[][] = { { 1 , 1 , 1 , 1 },
{ 2 , 2 , 2 , 2 },
{ 3 , 3 , 3 , 3 },
{ 4 , 4 , 4 , 4 } };
int B[][] = new int [N][N], i, j;
transpose(A, B);
System.out.print( "Result matrix is \n" );
for (i = 0 ; i < N; i++) {
for (j = 0 ; j < N; j++)
System.out.print(B[i][j] + " " );
System.out.print( "\n" );
}
}
}
|
Python3
N = 4
def transpose(A, B):
for i in range (N):
for j in range (N):
B[i][j] = A[j][i]
if __name__ = = '__main__' :
A = [[ 1 , 1 , 1 , 1 ],
[ 2 , 2 , 2 , 2 ],
[ 3 , 3 , 3 , 3 ],
[ 4 , 4 , 4 , 4 ]]
B = [[ 0 for x in range (N)] for y in range (N)]
transpose(A, B)
print ( "Result matrix is" )
for i in range (N):
for j in range (N):
print (B[i][j], " " , end = '')
print ()
|
C#
using System;
class GFG {
static int N = 4;
static void transpose( int [, ] A, int [, ] B)
{
int i, j;
for (i = 0; i < N; i++)
for (j = 0; j < N; j++)
B[i, j] = A[j, i];
}
public static void Main()
{
int [, ] A = { { 1, 1, 1, 1 },
{ 2, 2, 2, 2 },
{ 3, 3, 3, 3 },
{ 4, 4, 4, 4 } };
int [, ] B = new int [N, N];
transpose(A, B);
Console.WriteLine( "Result matrix is \n" );
for ( int i = 0; i < N; i++) {
for ( int j = 0; j < N; j++)
Console.Write(B[i, j] + " " );
Console.Write( "\n" );
}
}
}
|
Javascript
<script>
var N = 4;
function transpose(A , B) {
var i, j;
for (i = 0; i < N; i++)
for (j = 0; j < N; j++)
B[i][j] = A[j][i];
}
var A = [ [ 1, 1, 1, 1 ], [ 2, 2, 2, 2 ], [ 3, 3, 3, 3 ], [4, 4, 4, 4]];
var B = Array(N);
for (i=0;i<N;i++)
B[i] =Array(N).fill(0);
transpose(A, B);
document.write( "Result matrix is <br/>" );
for (i = 0; i < N; i++) {
for (j = 0; j < N; j++)
document.write(B[i][j] + " " );
document.write( "<br/>" );
}
</script>
|
PHP
<?php
function transpose(& $A , & $B )
{
$N = 4;
for ( $i = 0; $i < $N ; $i ++)
for ( $j = 0; $j < $N ; $j ++)
$B [ $i ][ $j ] = $A [ $j ][ $i ];
}
$A = array ( array (1, 1, 1, 1),
array (2, 2, 2, 2),
array (3, 3, 3, 3),
array (4, 4, 4, 4));
$N = 4;
transpose( $A , $B );
echo "Result matrix is \n" ;
for ( $i = 0; $i < $N ; $i ++)
{
for ( $j = 0; $j < $N ; $j ++)
{
echo $B [ $i ][ $j ];
echo " " ;
}
echo "\n" ;
}
?>
|
Output
Result matrix is
1 2 3 4
1 2 3 4
1 2 3 4
1 2 3 4
Time complexity: O(M x N).
Auxiliary Space: O(M x N), since M x N extra space has been used.
Program to find the transpose of a matrix using constant space:
Note – This approach works only for square matrices (i.e., – where no. of rows are equal to the number of columns). This algorithm is also known as an “in-place” algorithm as it uses no extra space to solve the problem.
Follow the given steps to solve the problem:
- Run a nested loop using two integer pointers i and j for 0 <= i < N and i+1 <= j < N
- Swap A[i][j] with A[j][i]
Below is the implementation of the above approach:
C++
#include <bits/stdc++.h>
using namespace std;
#define N 4
void transpose( int A[][N])
{
for ( int i = 0; i < N; i++)
for ( int j = i + 1; j < N; j++)
swap(A[i][j], A[j][i]);
}
int main()
{
int A[N][N] = { { 1, 1, 1, 1 },
{ 2, 2, 2, 2 },
{ 3, 3, 3, 3 },
{ 4, 4, 4, 4 } };
transpose(A);
printf ( "Modified matrix is \n" );
for ( int i = 0; i < N; i++) {
for ( int j = 0; j < N; j++)
printf ( "%d " , A[i][j]);
printf ( "\n" );
}
return 0;
}
|
Java
class GFG {
static final int N = 4 ;
static void transpose( int A[][])
{
for ( int i = 0 ; i < N; i++)
for ( int j = i + 1 ; j < N; j++) {
int temp = A[i][j];
A[i][j] = A[j][i];
A[j][i] = temp;
}
}
public static void main(String[] args)
{
int A[][] = { { 1 , 1 , 1 , 1 },
{ 2 , 2 , 2 , 2 },
{ 3 , 3 , 3 , 3 },
{ 4 , 4 , 4 , 4 } };
transpose(A);
System.out.print( "Modified matrix is \n" );
for ( int i = 0 ; i < N; i++) {
for ( int j = 0 ; j < N; j++)
System.out.print(A[i][j] + " " );
System.out.print( "\n" );
}
}
}
|
Python3
N = 4
def transpose(A):
for i in range (N):
for j in range (i + 1 , N):
A[i][j], A[j][i] = A[j][i], A[i][j]
A = [[ 1 , 1 , 1 , 1 ],
[ 2 , 2 , 2 , 2 ],
[ 3 , 3 , 3 , 3 ],
[ 4 , 4 , 4 , 4 ]]
transpose(A)
print ( "Modified matrix is" )
for i in range (N):
for j in range (N):
print (A[i][j], " " , end = '')
print ()
|
C#
using System;
class GFG {
static int N = 4;
static void transpose( int [, ] A)
{
for ( int i = 0; i < N; i++)
for ( int j = i + 1; j < N; j++) {
int temp = A[i, j];
A[i, j] = A[j, i];
A[j, i] = temp;
}
}
public static void Main()
{
int [, ] A = { { 1, 1, 1, 1 },
{ 2, 2, 2, 2 },
{ 3, 3, 3, 3 },
{ 4, 4, 4, 4 } };
transpose(A);
Console.WriteLine( "Modified matrix is " );
for ( int i = 0; i < N; i++) {
for ( int j = 0; j < N; j++)
Console.Write(A[i, j] + " " );
Console.WriteLine();
}
}
}
|
Javascript
<script>
var N = 4;
function transpose(A) {
for (i = 0; i < N; i++)
for (j = i + 1; j < N; j++) {
var temp = A[i][j];
A[i][j] = A[j][i];
A[j][i] = temp;
}
}
var A = [ [ 1, 1, 1, 1 ],
[ 2, 2, 2, 2 ],
[ 3, 3, 3, 3 ],
[ 4, 4, 4, 4 ] ];
transpose(A);
document.write( "Modified matrix is <br/>" );
for (i = 0; i < N; i++) {
for (j = 0; j < N; j++)
document.write(A[i][j] + " " );
document.write( "\<br/>" );
}
</script>
|
PHP
<?php
function transpose(& $A )
{
$N = 4;
for ( $i = 0; $i < $N ; $i ++)
for ( $j = $i + 1; $j < $N ; $j ++)
{
$temp = $A [ $i ][ $j ];
$A [ $i ][ $j ] = $A [ $j ][ $i ];
$A [ $j ][ $i ] = $temp ;
}
}
$N = 4;
$A = array ( array (1, 1, 1, 1),
array (2, 2, 2, 2),
array (3, 3, 3, 3),
array (4, 4, 4, 4));
transpose( $A );
echo "Modified matrix is " . "\n" ;
for ( $i = 0; $i < $N ; $i ++)
{
for ( $j = 0; $j < $N ; $j ++)
echo $A [ $i ][ $j ] . " " ;
echo "\n" ;
}
?>
|
Output
Modified matrix is
1 2 3 4
1 2 3 4
1 2 3 4
1 2 3 4
Time complexity: O(N2).
Auxiliary Space: O(1)
Like Article
Suggest improvement
Share your thoughts in the comments
Please Login to comment...